Using forEach instead of a "for" loop for easy iterations
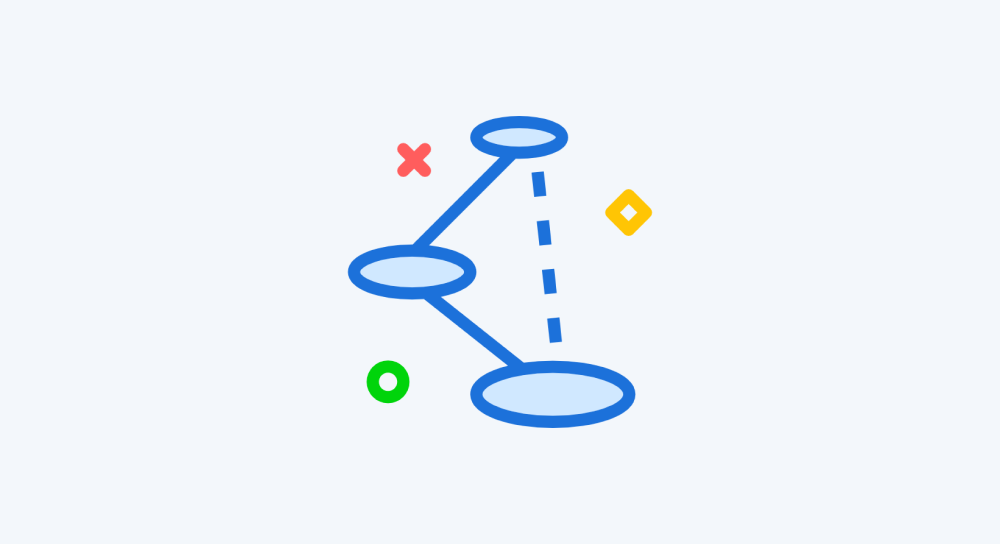
Now that you understand "for" loops in a detailed way, what if I tell you there is an easy shortcut to loop through each item inside an array?
When it comes to looping over an array with a "for" loop statement, you have to:
- Set up the counter
- Write a conditional statement that decides how many times a loop should run.
- Update the counter after every iteration.
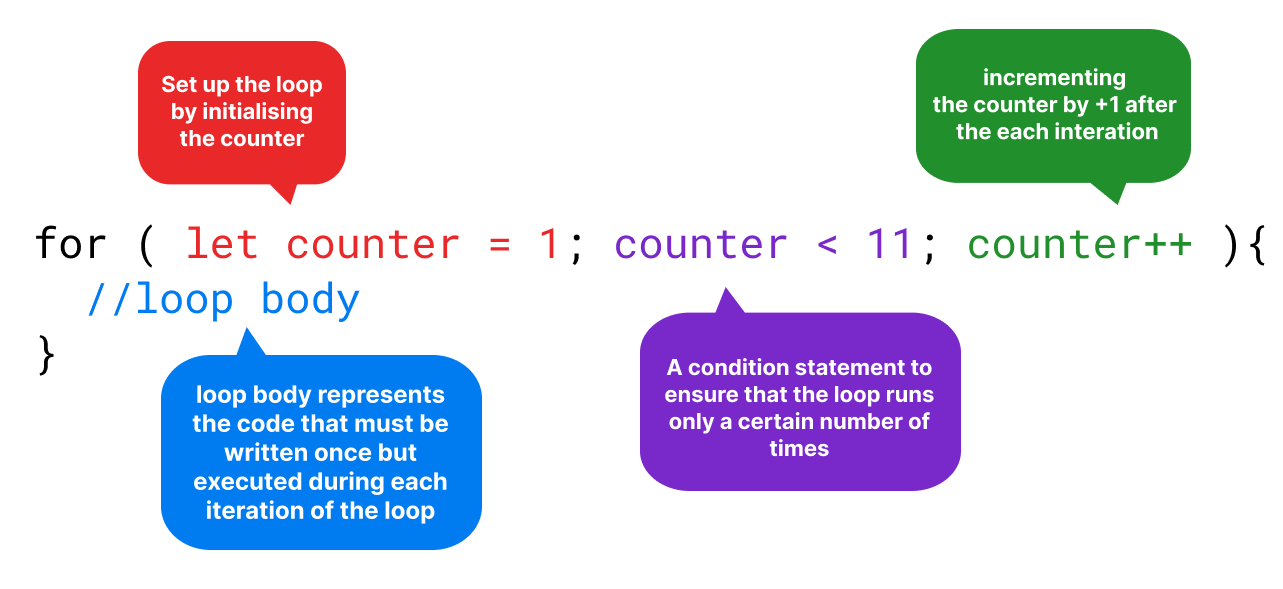
If we compare the "for" loop to cooking rice, writing a "for" loop is like cooking rice manually in three steps:
- Put the rice in a steel bowl and pour water in it
- Flame the stove and put the steel bowl with rice on top of the burner
- Then, you manually check if the rice is cooked or not after some time
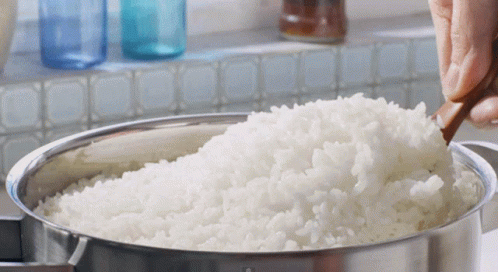
Introducing the forEach method
The forEach
method offers a shortcut for iterating over an array.
Just like push()
, pop()
, unshift()
, and shift()
methods that we have discussed so far, whenever you create an array, you get access to a method called forEach()
.
Here is a simple usage:
let unsafePasswords = ["12345", "qwerty123", "password"];
unsafePasswords.forEach( function(unsafePassword){
//Do something with each item inside the array
console.log(unSafePassword);
});
As you can see, unlike a "for" loop statement, if you're using the array.forEach()
method:
- You don't have to set up the counter and update it by yourself.
- You don't have to get the length of the array to set up the loop's conditional statement.
Instead:
arrayName.forEach(customFunction);
- You must call the
forEach
method on an array that you want to iterate over. - Next, to the forEach method, you must provide a custom function (callback) that contains instructions about what you want to do with each item inside the array.
- Then, the
forEach
method will automatically execute the custom function for each item inside the array.
Let me elaborate...
How forEach works
Before trying to understand the forEach method, first, let's understand a custom and manual version of it.
To do so, let's work on a small task where our goal is to manually access output all the items of an array into the browser console.
So, open up a new tab in the Chrome browser, open up its console, and put the following code in it:
//Step 1: Create an array
let fruits = ["Apple", "Banana", "Orange"];
//Step 2: Create a custom function that gets executed for each item inside the array
function printFruits(fruit){
console.log(fruit);
}
//Step 3: Call the custom above function for each item inside the array by providing the individual array item as an argument while calling the custom function.
printFruits(fruits[0]);
printFruits(fruits[1]);
printFruits(fruits[2]);
In the above code, first, we have created an array called fruits
:
let fruits = ["Apple", "Banana", "Orange"];
Then, we created a custom function called printFruits
whose only job is to output a fruit name to the browser console, and to achieve that, it expects a fruit name as the input parameter:
function printFruits(fruit){
console.log(fruit);
}
When this function is executed, the provided fruit name will be outputted to the browser console.
Finally, to achieve our goal of manually outputting each item from the fruits
array, all we have to do is call the printFruits
function for each item of the fruits
array:
printFruits(fruits[0]); //Outputs: Apple
printFruits(fruits[1]); //Outputs: Banana
printFruits(fruits[2]); //Outputs: Orange
There are three items inside the fruits
array, so I have called the printFruits
function three items.
While calling the printFruits
function for each item, I have provided the individual fruit names by accessing them from the array.
And this results in the following output:
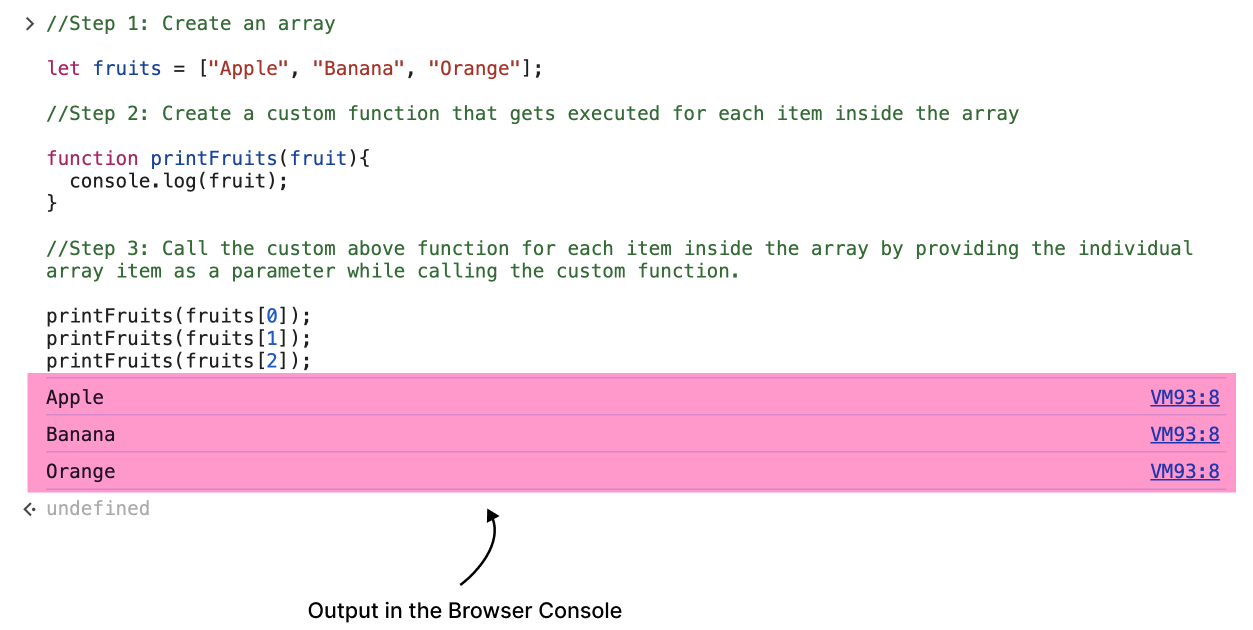
Easy, so far?
And when you call the forEach()
method on an array, it behaves exactly like, but it calls the printFruits
function automatically for each item of the fruits
array:
//Step 1: Create an array
let fruits = ["Apple", "Banana", "Orange"];
//Step 2: Create a custom function that gets executed for each item inside the array
function printFruits(fruit){
console.log(fruit);
}
//Step 3: Call the custom above function for each item inside the array by providing the individual array item as an argument while calling the custom function.
fruits.forEach(printFruits);
Simply put, the following code:
printFruits(fruits[0]); //Outputs: Apple
printFruits(fruits[1]); //Outputs: Banana
printFruits(fruits[2]); //Outputs: Orange
Is the same as:
fruits.forEach(printFruits);
When going with the manual route, we are manually accessing each item from the fruits
array and then feeding them to the printFruits
function manually:
printFruits(fruits[0]); //Outputs: Apple
printFruits(fruits[1]); //Outputs: Banana
printFruits(fruits[2]); //Outputs: Orange
But when using the forEach
method, while calling the printFruits function, we don't have to do the manual work:
fruits.forEach(printFruits);
Simply put, we don't have to manually access each item from the fruits
array and then feeding it to the printFruits
function manually.
The forEach()
method does it for you automatically.
So, coming back to our rice analogy, the forEach
method is more like an automatic rice cooker:
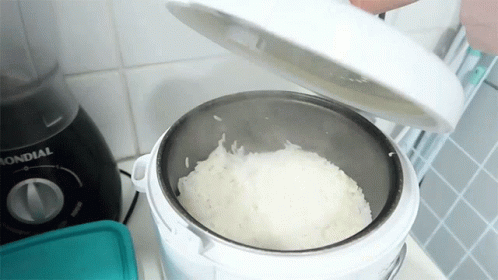
Anyway, just to be on the same page, let's see what's happening for each iteration when we use the forEach
method on the fruits
array.
During iteration 1:
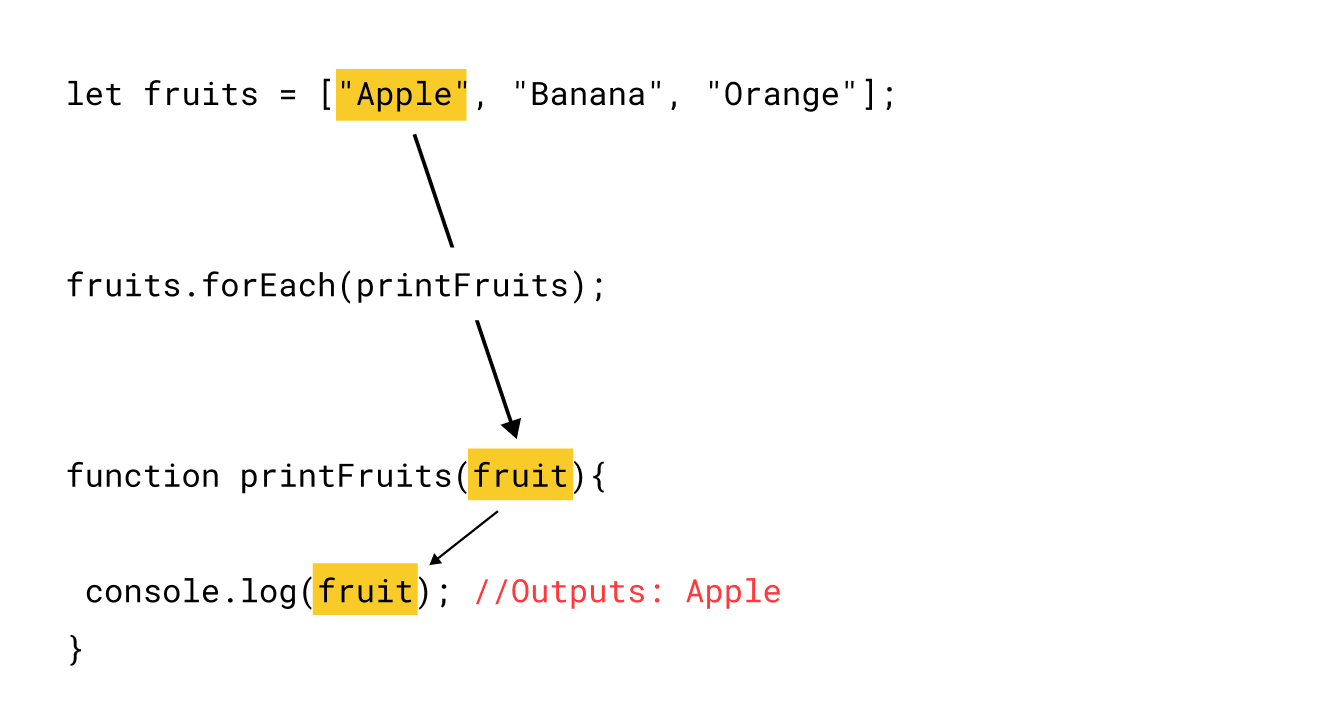
- The
forEach()
method picks the first item from thefruits
array, that is"Apple"
- Next, it calls the
printFruits
function automatically by providing"Apple"
as an argument to it. - Finally, the
printFruits
function outputs "Apple" to the console.
During iteration 2:
- The
forEach()
method picks the second item from thefruits
array, that is"Banana"
- Next, it calls the
printFruits
function automatically by providing"Banana"
as an argument to it. - Finally, the
printFruits
function outputs "Banana" to the console.
During iteration 3:
- The
forEach()
method picks the second item from thefruits
array, that is"Orange"
- Next, it calls the
printFruits
function automatically by providing"Orange"
as an argument to it. - Finally, the
printFruits
function outputs "Orange" to the console.
The forEach() method will continue the process until it runs out of all the items from the array.
In our current case, there are only three items inside the fruits
array, so the forEach()
method will stop calling the printFruits
function after the third iteration because there are no more items left.
Anyway, in the next lesson, we will talk about how we can convert the printFruits
into an anonymous function and feed it to the forEach()
method.