Using an anonymous function with the forEach method
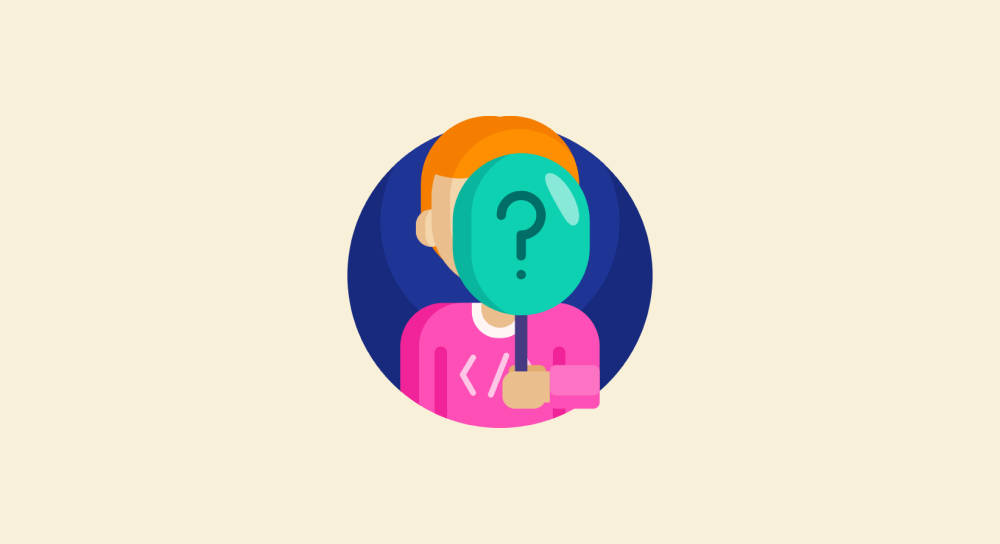
Here is where we are at during the last lesson:
let fruits = ["Apple", "Banana", "Orange"];
function printFruits(fruit){
console.log(fruit);
}
fruits.forEach(printFruits);
In the above code, we created a custom function called printFruits
, and then we are providing its name as an argument to the forEach()
method.
When it comes to terminology, you can also call the creation of a function as a function definition.
Now, the thing is, instead of just providing a function name to the forEach()
method, we can also provide the function definition as an argument directly:
let fruits = ["Apple", "Banana", "Orange"];
fruits.forEach(function printFruits(fruit){
console.log(fruit);
});
This will work in exactly the same way and outcome of the forEach()
method still remains the same because, at the end of the day, the forEach()
method needs a function reference to call it on each item.
And in Javascript, you can provide a function reference either by providing the function name:
let fruits = ["Apple", "Banana", "Orange"];
function printFruits(fruit){
console.log(fruit);
}
fruits.forEach(printFruits);
or its function "definition" directly:
let fruits = ["Apple", "Banana", "Orange"];
fruits.forEach(function printFruits(fruit){
console.log(fruit);
});
Having said that, we can go a step further and make the function definition an anonymous function definition because we are not calling the printFruits
function using its name by ourselves (manually).
Instead, the forEach()
method is doing it automatically for us.
So, we can rewrite the above code as:
let fruits = ["Apple", "Banana", "Orange"];
fruits.forEach(function(fruit){
console.log(fruit);
});
In the above code, we just removed the name printFruits
from the function definition and made it an anonymous function.
This makes the code more readable and concise because everything is in one place.
If you remember, we did the same thing by using an anonymous function to handle events.
First, we started off using a named function to handle the event:
function showModal(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
}
let showModalButton = document.querySelector(".show-modal-btn");
showModalButton.addEventListener("click", showModal);
But then, when we realized that we could provide a function definition directly as the event handler, we started using an anonymous function for its conciseness and better readability:
showModalButton.addEventListener("click", function(){
let discountModal = document.querySelector("#surprise-discount-modal");
discountModal.style.display = "block";
});
And we did the same thing with forEach
method as well.
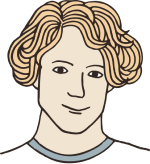
All this is good. But bro, I am confused about when to use a named function and an anonymous function.
My bad. I should have introduced the concept of callback functions so that you can clearly take your function-related decisions.
But it's not too late. Let's discuss callback functions in the next lesson.