Understanding Lists in Programming
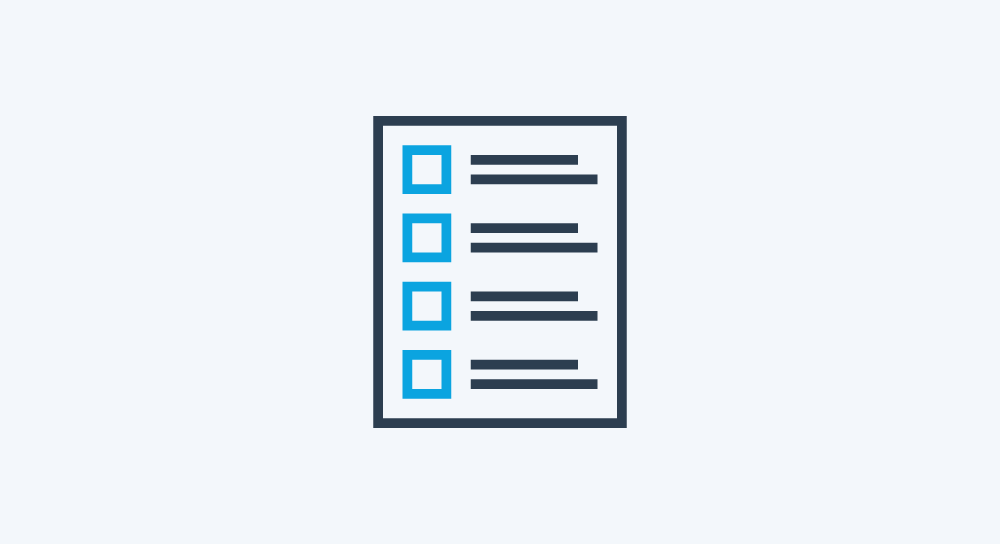
In programming, we often have to deal with lists.
So, what is a list?
A list is nothing but a collection of items or data.
For example, a list could be a collection of music albums.
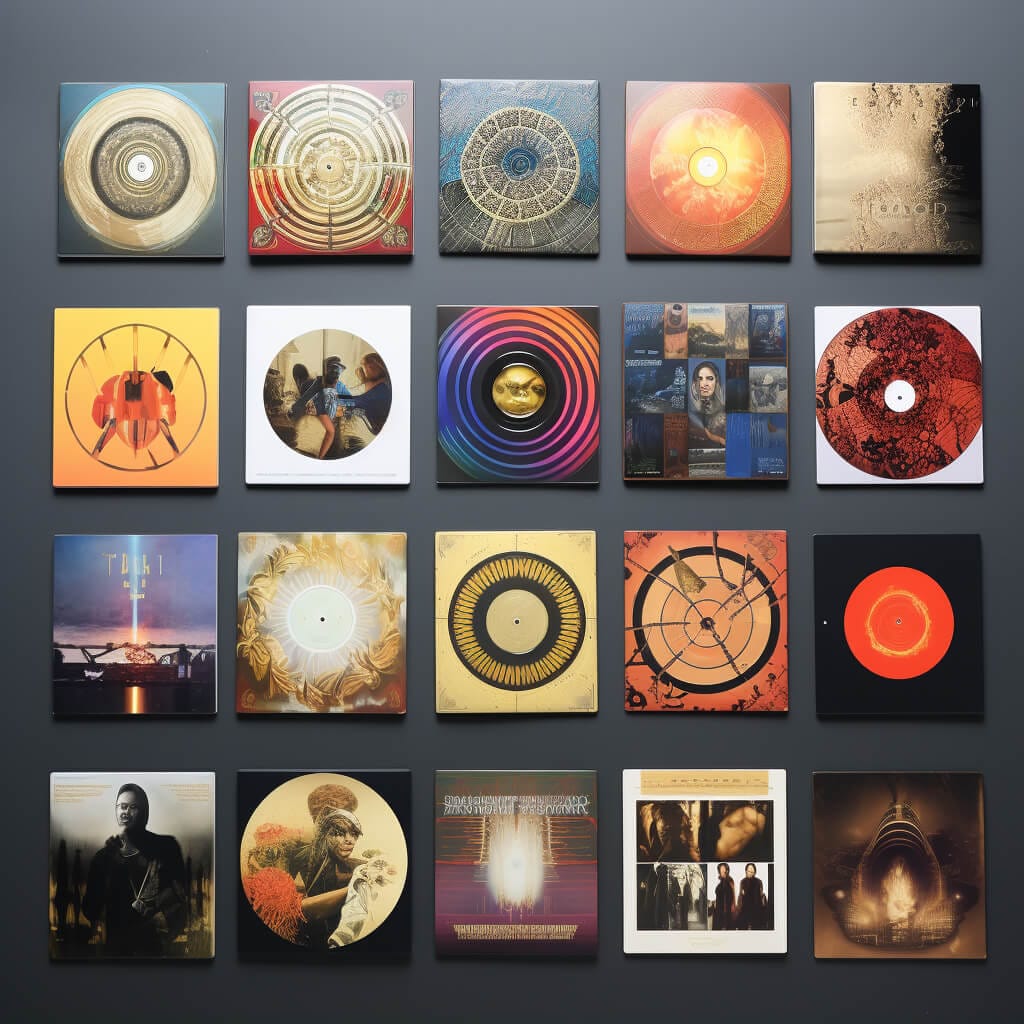
Or a list of items that you want to buy in a Supermarket:
- Cereals & Museli
- Apples
- Coffee
- Fruit juice
- Energy Drink
- Broccoli
Or a list of names that you're considering for your kid 🕶️
You get the idea, right?
These are real-world lists that we are closely connected to.
But sooner or later, you have to deal with these lists in your coding projects, too.
For example, if you're creating a music app like Spotify, you must mimic a music album list using the code:
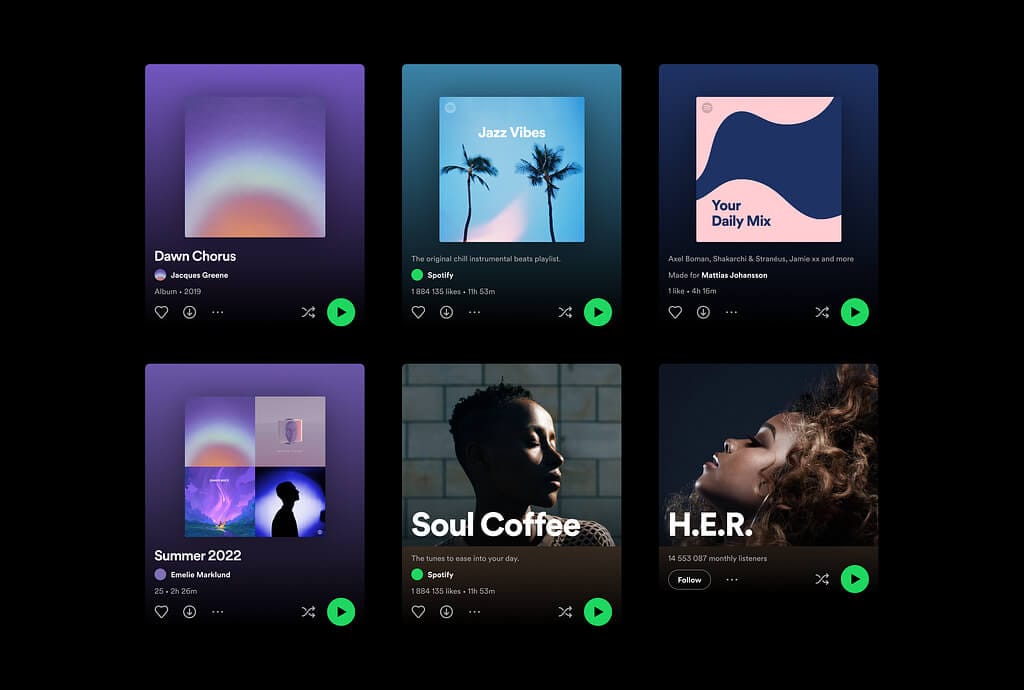
Similarly, if you're building an e-commerce store, you have work with variety of lists such as:
1) Shopping cart list
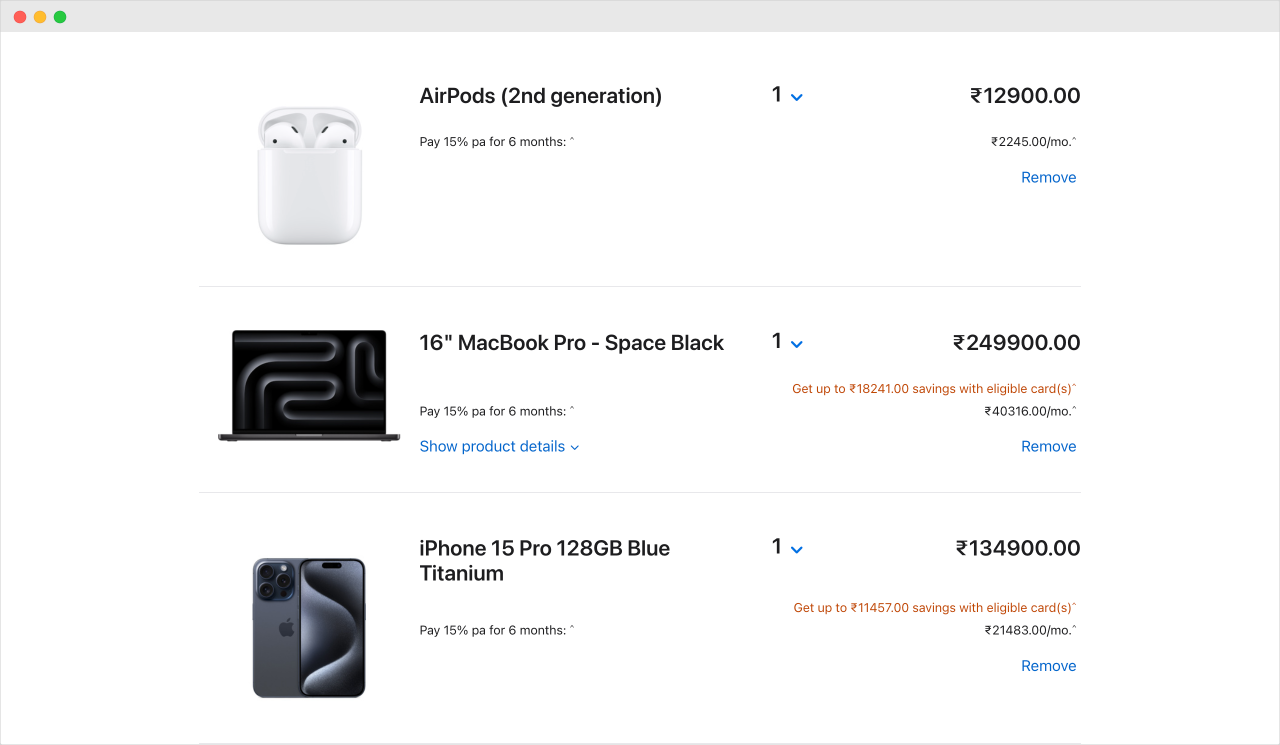
2) Product catalog and product category lists:
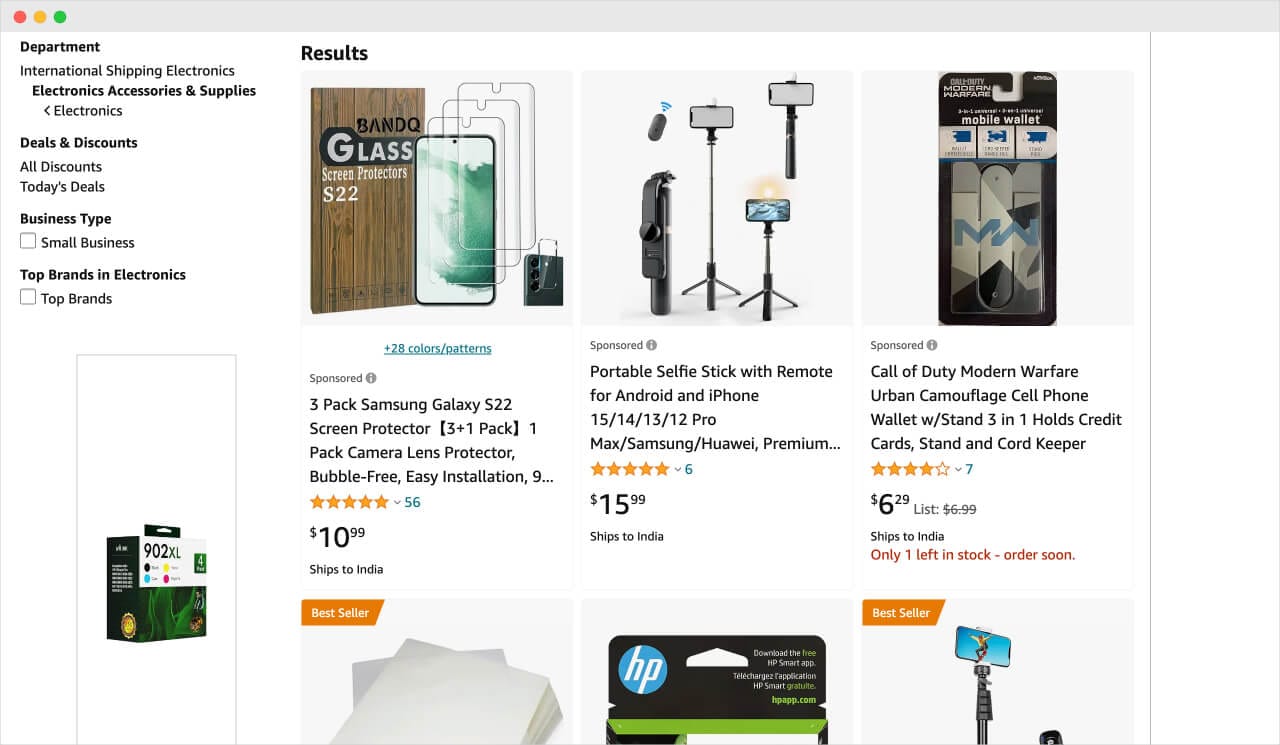
Or a list of unsafe passwords to reject in an account registration form:
- admin
- yourname@123
- 123456
Long story short, when coding in Javascript, dealing with a list of data becomes inevitable.
This brings us to an important question: How do we create and manage these lists in Javascript?
So far, we have been creating only a single piece of data:
const name = "Naresh Devineni"; // String
let age = 34; // Number
let isMarried = false; // Boolean
let hasRemarks = null; // Null
If you notice the above snippet, all four individual pieces of data are of a single value.
Sure, we have seen how to create a more complex piece of data using an object:
let iPhone = {
brandName: "Apple", // String
cardSlot: false, // Boolean
price: 1200, // Number
headPhoneJack: null, // Null value
misc: { // Object
colors: ["black","red", "space grey" ], // Array (more on this later)
batterLife: "62 Hours", // String
models: ["A2783", "A2595", "A2785"] // Array
}
output_properties_as_table: function() {} //Function (more on this later)
};
An iPhone is represented as an object
But, an object still represents a single entity.
In the above example, the object represents data about a single iPhone only. Not multiple iPhones.
So, how do we create a list of data?
For starters, we can create lists using the following two features of Javascript:
- Array - It allows us to create and manage a standard list with literal data, such as a list of countries, shopping cart items, product lists, questions for the quiz, etc.
- NodeList - It is a list of HTML elements such as FAQs, gallery thumbnails, Add-to-cart buttons on a shop page, etc. A NodeList is created when selecting HTML elements using Javascript.
1) Arrays
Arrays are the most common way to create standard lists in JavaScript.
Sometimes, you'll manually create an array by literally typing a list of data, such as a list of unsafe passwords:
let unsafePasswords = ["123456", "admin", "yourname@123", "password"];
Or a list of countries:
let countriesList = ["India", "Pakistan", "Bangladesh", "China"];
But sometimes, you'll get an array with dynamic data as a result of making a function call:
let activeUsers = getUsersWithSubscriptions();
In the above snippet, getUsersWithSubscription()
function call communicates with a database and returns the user list as an array.
You can't type the list of dynamic users manually by your hand. It has to be a result of some database query.
More on this in a future module. Anyway...
An array also allows you to store a list of items in an organized way.
Each item inside an array will have a unique index, such as 0, 1, 2, 3, etc.
Because of this, once an array is created, we can:
- Retrieve any item or multiple items from the list
- Delete an item or multiple items from the list
- Add an item or multiple items to the list
- Change the order of the items in the list
- Iterate through each item in the list to do something with them. For example, outputting them on a web page.
As you can see, arrays are organized, flexible, and dynamic. So, they are suitable for various tasks in a programmer's day-to-day life.
Also, the items in the list can be of any data type—more on this in the next lesson.
The next common type of list we can create is a NodeList.
2) NodeLists
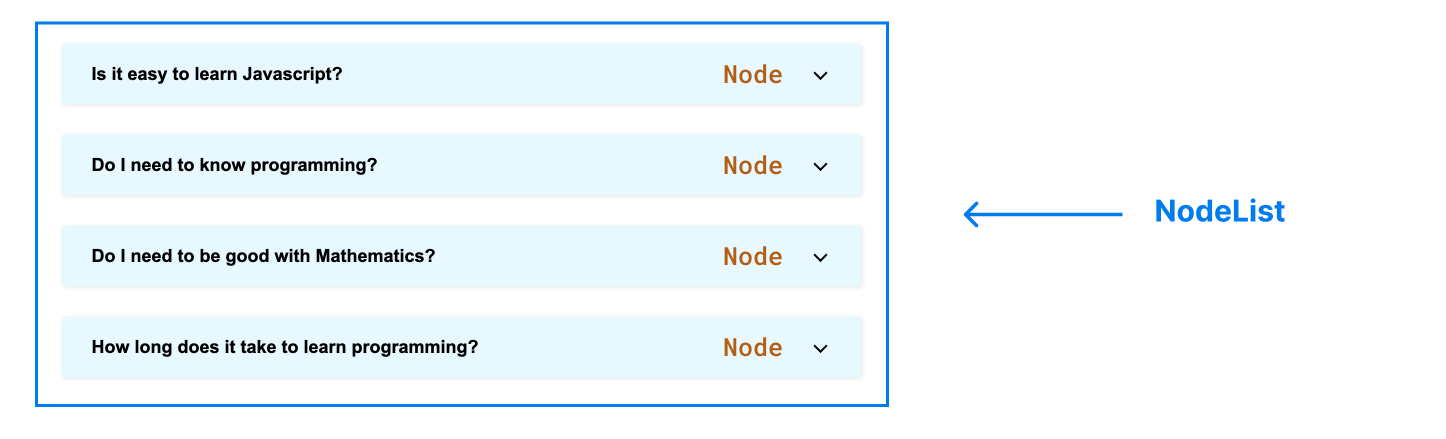
A NodeList is nothing but a collection of selected HTML elements from a web page.
A NodeList is created when we select multiple HTML elements using the document.querySelectorAll()
method or similar methods.
For example:
let faqAccordions = document.querySelectAll(".faqs .accordion");
The above line of code returns a collection of HTML elements that match the .accordion
CSS selector.
And just like Arrays, Once a NodeList is created, we can:
- Retrieve a single HTML element or multiple elements from the NodeList
- Delete an HTML element or multiple elements from the NodeList
- Add an HTML element or multiple elements to the NodeList
- Change the order of the HTML elements. For example, showing a dictionary of definitions from Z - A instead of A - Z.
- Iterate through each HTML element in the NodeList to do something with them. For example, add an event listener to make them clickable.
This also means...
If you learn how to manage an Array, you know how to manage a NodeList or any other type of list in Javascript.
So, starting from the next lesson, let's understand Arrays in a deeper way. It will help us work with other lists in Javascript in an easy way.