The need for loops
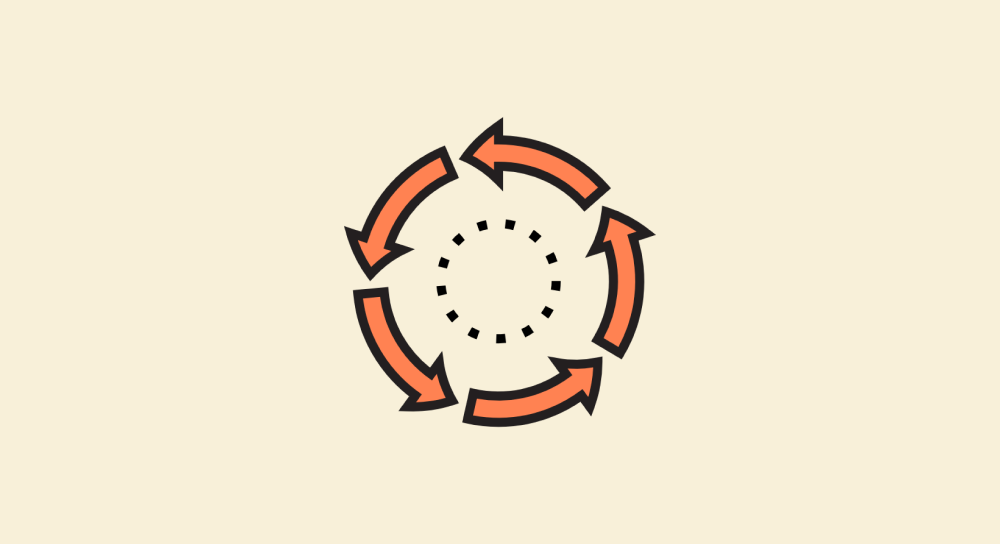
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
//Select the parent element
const olElement = document.querySelector("#unsafe-passwords");
// Outputting the first item in to the web page
const firstLiElement = document.createElement("li");
firstLiElement.textContent = unsafePasswords[0];
olElement.appendChild(firstLiElement);
// Outputting the second item in to the web page
const secondLiElement = document.createElement("li");
secondLiElement.textContent = unsafePasswords[1];
olElement.appendChild(secondLiElement);
// Outputting the third item in to the web page
const thirdLiElement = document.createElement("li");
thirdLiElement.textContent = unsafePasswords[2];
olElement.appendChild(thirdLiElement);
// Outputting the fourth item in to the web page
const fourthLiElement = document.createElement("li");
fourthLiElement.textContent = unsafePasswords[3];
olElement.appendChild(fourthLiElement);
// Outputting the fifth item in to the web page
const fifthLiElement = document.createElement("li");
fifthLiElement.textContent = unsafePasswords[4];
olElement.appendChild(fifthLiElement);
Did you find any inconvenience with the above code we have written during the previous exercise?
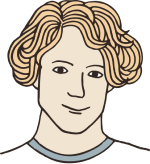
Not sure 🙈
Okay!
The problem is we have written a lot of repetitive code
If you notice the above snippet:
1) We have written a set of codes to access the first unsafe password from the array and then displayed it on the page.
// Outputting the first item in to the web page
const firstLiElement = document.createElement("li");
firstLiElement.textContent = unsafePasswords[0];
olElement.appendChild(firstLiElement);
2) Then, we repeated that exact code a second time, a third time, etc., until we displayed all the unsafe passwords from an array.
// Outputting the second item in to the web page
const secondLiElement = document.createElement("li");
secondLiElement.textContent = unsafePasswords[1];
olElement.appendChild(secondLiElement);
// Outputting the third item in to the web page
const thirdLiElement = document.createElement("li");
thirdLiElement.textContent = unsafePasswords[2];
olElement.appendChild(thirdLiElement);
// Outputting the fourth item in to the web page
const fourthLiElement = document.createElement("li");
fourthLiElement.textContent = unsafePasswords[3];
olElement.appendChild(fourthLiElement);
// Outputting the fifth item in to the web page
const fifthLiElement = document.createElement("li");
fifthLiElement.textContent = unsafePasswords[4];
olElement.appendChild(fifthLiElement);
Simply put, we are repeating the same set of codes for outputting each password from the array.
Only the variable names and array index values are changing. Everything else is the same.
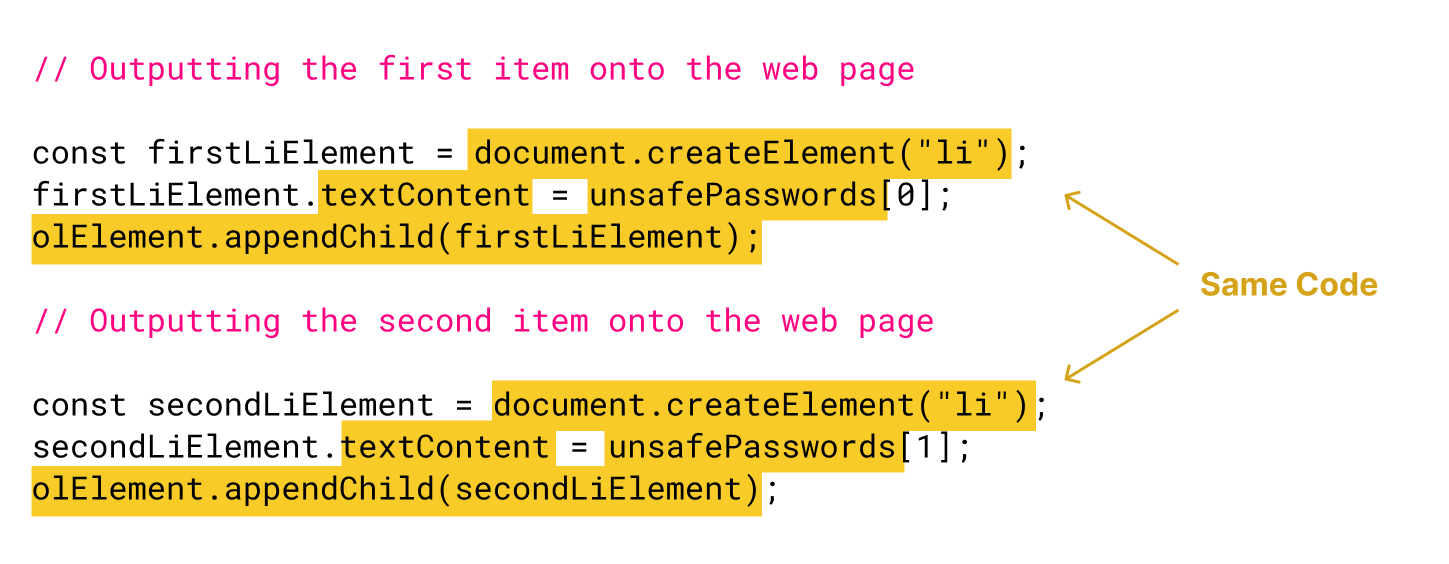
This repetition makes the code longer than necessary and more challenging to maintain. This, in turn, leads to errors.
This is more than a common scenario; most importantly, it violates the DRY principle (Don't Repeat Yourself) in programming.
In the above snippet, we outputted only five passwords onto the web page.
But what if you want to output twenty passwords?
Would you keep writing the same set of codes for outputting each of those 20 passwords?
If you do so, here is how long the code looks:
let unsafePasswords = [
"123456",
"123456789",
"12345",
"qwerty",
"password",
"12345678",
"111111",
"123123",
"1234567890",
"1234567",
"qwerty123",
"000000",
"1q2w3e",
"aa12345678",
"abc123",
"password1",
"1234",
"qwertyuiop",
"123321",
"password123"
];
//Select the parent element
const olElement = document.querySelector("#unsafe-passwords");
// Outputting the first item in to the web page
const firstLiElement = document.createElement("li");
firstLiElement.textContent = unsafePasswords[0];
olElement.appendChild(firstLiElement);
// Outputting the second item in to the web page
const secondLiElement = document.createElement("li");
secondLiElement.textContent = unsafePasswords[1];
olElement.appendChild(secondLiElement);
// Outputting the third item in to the web page
const thirdLiElement = document.createElement("li");
thirdLiElement.textContent = unsafePasswords[2];
olElement.appendChild(thirdLiElement);
// Outputting the fourth item in to the web page
const fourthLiElement = document.createElement("li");
fourthLiElement.textContent = unsafePasswords[3];
olElement.appendChild(fourthLiElement);
// Outputting the fifth item in to the web page
const fifthLiElement = document.createElement("li");
fifthLiElement.textContent = unsafePasswords[4];
olElement.appendChild(fifthLiElement);
// Outputting the sixth item in to the web page
const sixthLiElement = document.createElement("li");
sixthLiElement.textContent = unsafePasswords[5];
olElement.appendChild(sixthLiElement);
// Outputting the seventh item in to the web page
const seventhLiElement = document.createElement("li");
seventhLiElement.textContent = unsafePasswords[6];
olElement.appendChild(seventhLiElement);
// Outputting the eighth item in to the web page
const eighthLiElement = document.createElement("li");
eighthLiElement.textContent = unsafePasswords[7];
olElement.appendChild(eighthLiElement);
// Outputting the ninth item in to the web page
const ninthLiElement = document.createElement("li");
ninthLiElement.textContent = unsafePasswords[8];
olElement.appendChild(ninthLiElement);
// Outputting the tenth item in to the web page
const tenthLiElement = document.createElement("li");
tenthLiElement.textContent = unsafePasswords[9];
olElement.appendChild(tenthLiElement);
// Outputting the eleventh item in to the web page
const eleventhLiElement = document.createElement("li");
eleventhLiElement.textContent = unsafePasswords[10];
olElement.appendChild(eleventhLiElement);
// Outputting the twelfth item in to the web page
const twelfthLiElement = document.createElement("li");
eleventhLiElement.textContent = unsafePasswords[11];
olElement.appendChild(twelfthLiElement);
// Outputting the thirteenth item in to the web page
const thirteenthLiElement = document.createElement("li");
thirteenthLiElement.textContent = unsafePasswords[12];
olElement.appendChild(thirteenthLiElement);
// Outputting the fourteenth item in to the web page
const fourteenthLiElement = document.createElement("li");
fourteenthLiElement.textContent = unsafePasswords[13];
olElement.appendChild(fourteenthLiElement);
// Outputting the fifteenth item in to the web page
const fifteenthLiElement = document.createElement("li");
fifteenthLiElement.textContent = unsafePasswords[14];
olElement.appendChild(fifteenthLiElement);
// Outputting the sixteenth item in to the web page
const sixteenthLiElement = document.createElement("li");
sixteenthLiElement.textContent = unsafePasswords[15];
olElement.appendChild(sixteenthLiElement);
// Outputting the seventeenth item in to the web page
const seventeenthLiElement = document.createElement("li");
seventeenthLiElement.textContent = unsafePasswords[16];
olElement.appendChild(seventeenthLiElement);
// Outputting the eighteenth item in to the web page
const eighteenthLiElement = document.createElement("li");
eighteenthLiElement.textContent = unsafePasswords[17];
olElement.appendChild(eighteenthLiElement);
// Outputting the nineteenth item in to the web page
const nineteenthLiElement = document.createElement("li");
nineteenthLiElement.textContent = unsafePasswords[18];
olElement.appendChild(nineteenthLiElement);
// Outputting the twentieth item in to the web page
const twentiethLiElement = document.createElement("li");
twentiethLiElement.textContent = unsafePasswords[19];
olElement.appendChild(twentiethLiElement);
Do you see the problem now?
Now, what if there are 40 passwords to output? 🤣
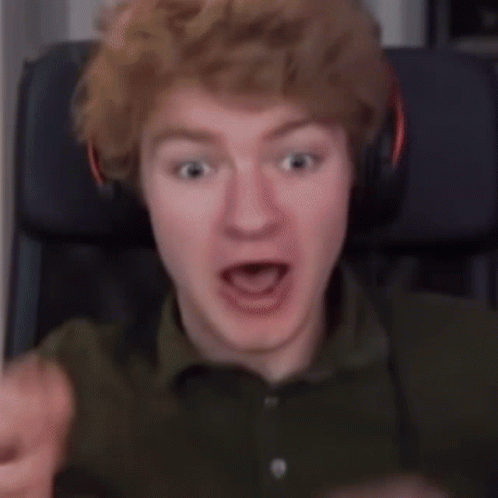
By the way, there is a small problem in the above code.
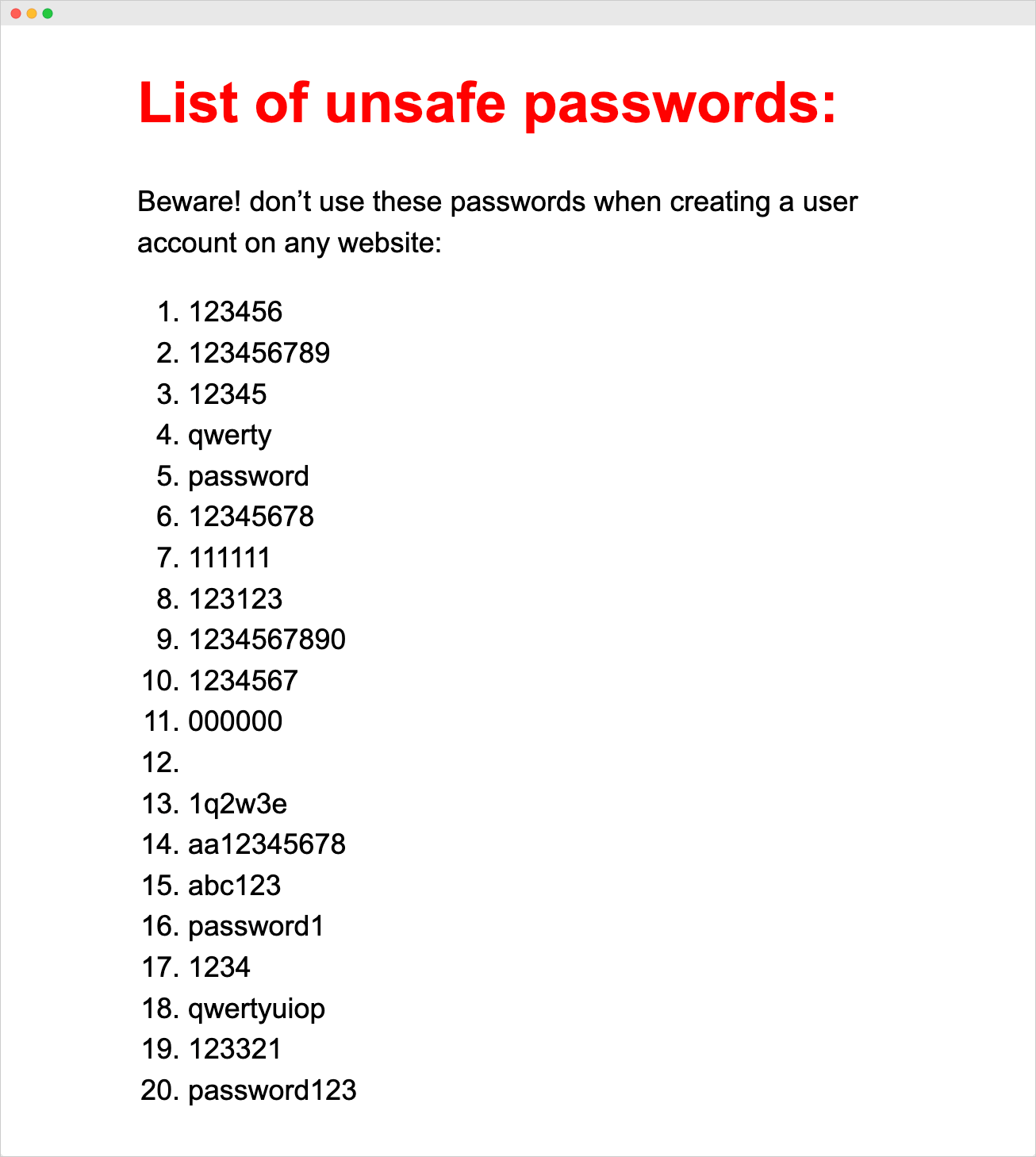
For some reason, one of the passwords is not getting outputted.
Go figure out why it is missing.
Did you figure it out?
...
...
...
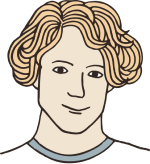
Yeah Bro! There is a variable name mismatch. It took me some time to skim through the code, though.
Yeah, and this is what I meant when I said that:
"This repetition makes the code longer than necessary and harder to maintain. This, in turn, leads to errors."
The above "long & repetitive" code is just like a "long & open" running track that you're building to conduct a 10000-meter race:
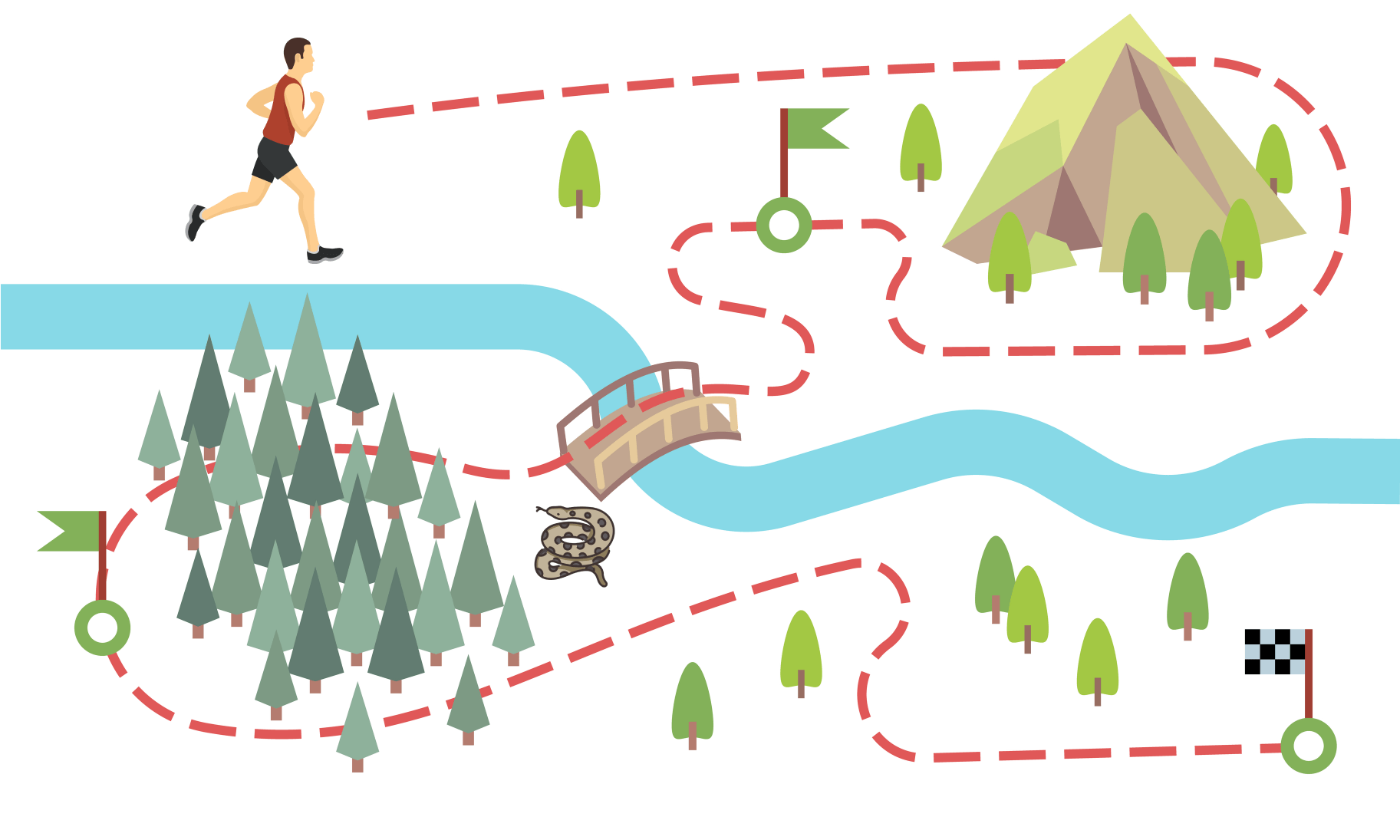
Just like our long code, the above running track takes a long time to build, is harder to maintain, and puts the runners at risk of road accidents, dangerous animals wandering around, etc.
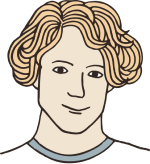
Now I got it! So, what is the solution here?
Simple...
We have to automate it using loops
A loop simply means repeating an action a certain number of times or until a condition is met.
In other words, Loops are a way to repeat the same code multiple times.
For example, an action could be picking an item from the array and displaying it on the web page.
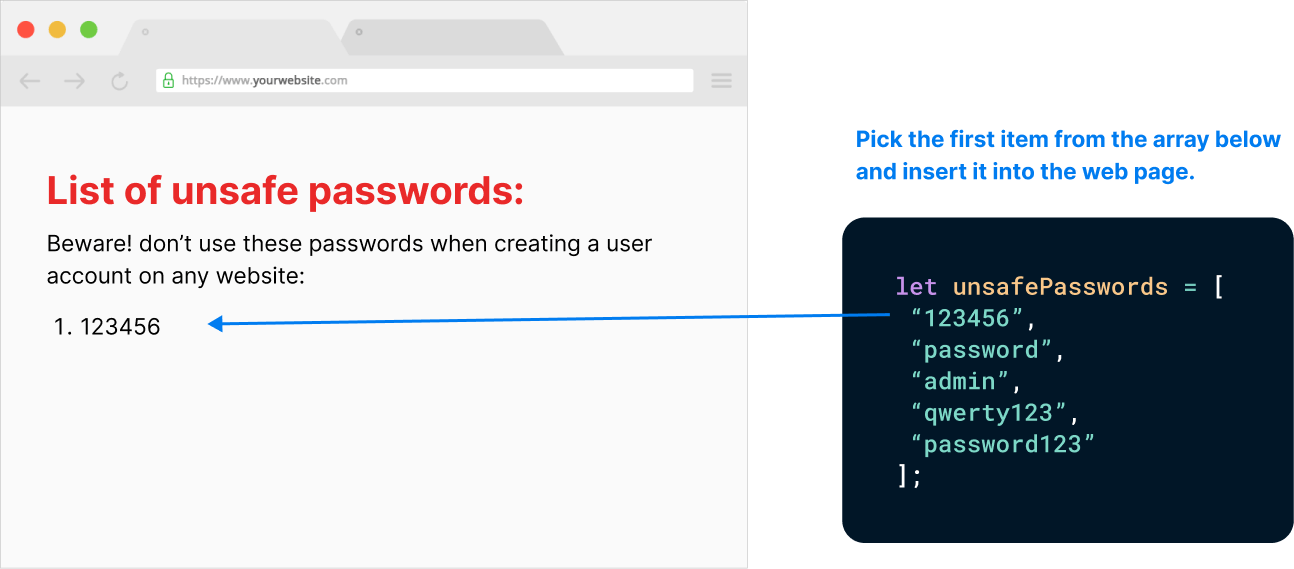
Repeating an action a certain number of times means picking each item from the array repeatedly and displaying them on the web page until we have displayed all the items from the array.
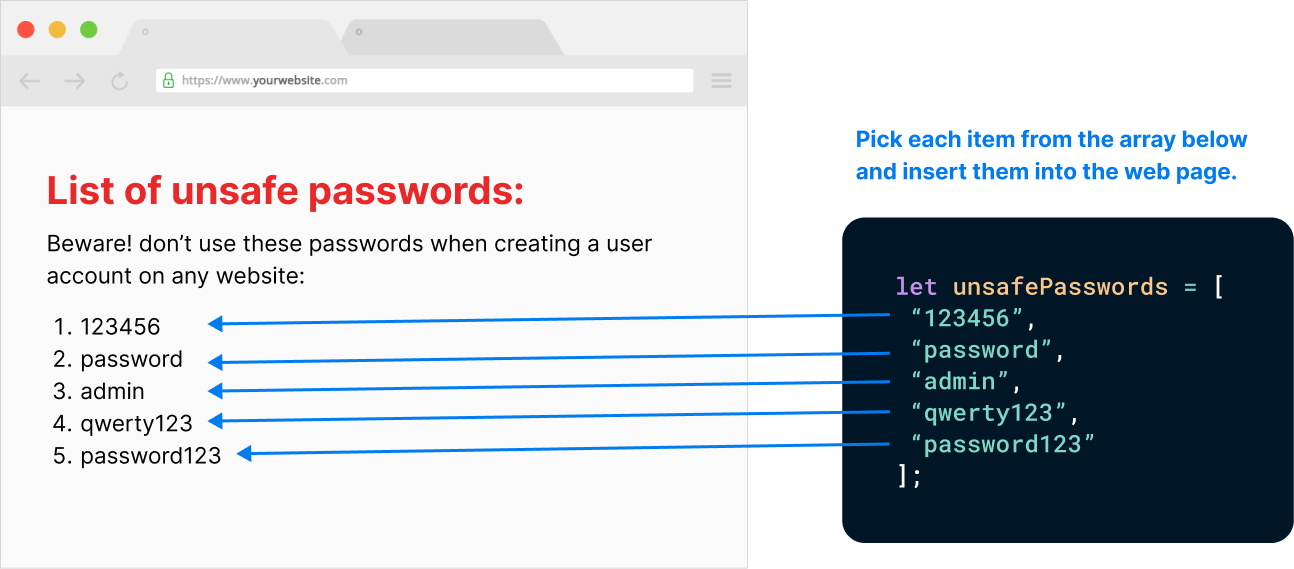
We are already doing this with our long, repetitive code.
But by using a "loop statement" in programming, we can automate it.
When using a loop statement:
- The action is implemented by writing a code once
- Then, have the Javascript engine execute that code ten times, forty times, or as many times as you want.
Javascript provides us with many loop statements, but the most popular is the "for" loop statement.
For example, for our use case of displaying twenty unsafe passwords on a web page, here is how we can replace the long repetitive code with a simple "for" loop:
let unsafePasswords = [
"123456",
"123456789",
"12345",
"qwerty",
"password",
"12345678",
"111111",
"123123",
"1234567890",
"1234567",
"qwerty123",
"000000",
"1q2w3e",
"aa12345678",
"abc123",
"password1",
"1234",
"qwertyuiop",
"123321",
"password123"
];
//Select the parent element
const olElement = document.querySelector("#unsafe-passwords");
//The "for" loop that avoids the long repetitive code
for(let i = 0; i < unsafePasswords.length; i++){
const liElement = document.createElement("li");
liElement.textContent = unsafePasswords[i];
olElement.appendChild(liElement);
}
Don't worry about understanding the above code yet!
Without repeating the code for each item, the "for" loop statement in the above code loops through all 20 items in the array and outputs each item to the web page automatically:
//The "for" loop that is executing same code multiple times
for(let i = 0; i < unsafePasswords.length; i++){
const liElement = document.createElement("li");
liElement.textContent = unsafePasswords[i];
olElement.appendChild(liElement);
}
In other words, the above "five lines" of loop code do the same job as the following "99 lines" of repetitive code:
// Outputting the first item in to the web page
const firstLiElement = document.createElement("li");
firstLiElement.textContent = unsafePasswords[0];
olElement.appendChild(firstLiElement);
// Outputting the second item in to the web page
const secondLiElement = document.createElement("li");
secondLiElement.textContent = unsafePasswords[1];
olElement.appendChild(secondLiElement);
// Outputting the third item in to the web page
const thirdLiElement = document.createElement("li");
thirdLiElement.textContent = unsafePasswords[2];
olElement.appendChild(thirdLiElement);
// Outputting the fourth item in to the web page
const fourthLiElement = document.createElement("li");
fourthLiElement.textContent = unsafePasswords[3];
olElement.appendChild(fourthLiElement);
// Outputting the fifth item in to the web page
const fifthLiElement = document.createElement("li");
fifthLiElement.textContent = unsafePasswords[4];
olElement.appendChild(fifthLiElement);
// Outputting the sixth item in to the web page
const sixthLiElement = document.createElement("li");
sixthLiElement.textContent = unsafePasswords[5];
olElement.appendChild(sixthLiElement);
// Outputting the seventh item in to the web page
const seventhLiElement = document.createElement("li");
seventhLiElement.textContent = unsafePasswords[6];
olElement.appendChild(seventhLiElement);
// Outputting the eighth item in to the web page
const eighthLiElement = document.createElement("li");
eighthLiElement.textContent = unsafePasswords[7];
olElement.appendChild(eighthLiElement);
// Outputting the ninth item in to the web page
const ninthLiElement = document.createElement("li");
ninthLiElement.textContent = unsafePasswords[8];
olElement.appendChild(ninthLiElement);
// Outputting the tenth item in to the web page
const tenthLiElement = document.createElement("li");
tenthLiElement.textContent = unsafePasswords[9];
olElement.appendChild(tenthLiElement);
// Outputting the eleventh item in to the web page
const eleventhLiElement = document.createElement("li");
eleventhLiElement.textContent = unsafePasswords[10];
olElement.appendChild(eleventhLiElement);
// Outputting the twelfth item in to the web page
const twelfthLiElement = document.createElement("li");
eleventhLiElement.textContent = unsafePasswords[11];
olElement.appendChild(twelfthLiElement);
// Outputting the thirteenth item in to the web page
const thirteenthLiElement = document.createElement("li");
thirteenthLiElement.textContent = unsafePasswords[12];
olElement.appendChild(thirteenthLiElement);
// Outputting the fourteenth item in to the web page
const fourteenthLiElement = document.createElement("li");
fourteenthLiElement.textContent = unsafePasswords[13];
olElement.appendChild(fourteenthLiElement);
// Outputting the fifteenth item in to the web page
const fifteenthLiElement = document.createElement("li");
fifteenthLiElement.textContent = unsafePasswords[14];
olElement.appendChild(fifteenthLiElement);
// Outputting the sixteenth item in to the web page
const sixteenthLiElement = document.createElement("li");
sixteenthLiElement.textContent = unsafePasswords[15];
olElement.appendChild(sixteenthLiElement);
// Outputting the seventeenth item in to the web page
const seventeenthLiElement = document.createElement("li");
seventeenthLiElement.textContent = unsafePasswords[16];
olElement.appendChild(seventeenthLiElement);
// Outputting the eighteenth item in to the web page
const eighteenthLiElement = document.createElement("li");
eighteenthLiElement.textContent = unsafePasswords[17];
olElement.appendChild(eighteenthLiElement);
// Outputting the nineteenth item in to the web page
const nineteenthLiElement = document.createElement("li");
nineteenthLiElement.textContent = unsafePasswords[18];
olElement.appendChild(nineteenthLiElement);
// Outputting the twentieth item in to the web page
const twentiethLiElement = document.createElement("li");
twentiethLiElement.textContent = unsafePasswords[19];
olElement.appendChild(twentiethLiElement);
Huge difference, right?
That's how a simple loop implementation makes our life easier as a programmer.
If we compare the "long & repetitive code" to a "10000 meters wild open running track", then we can compare the "for" loop statement to a small 1000 meters running track in a stadium:
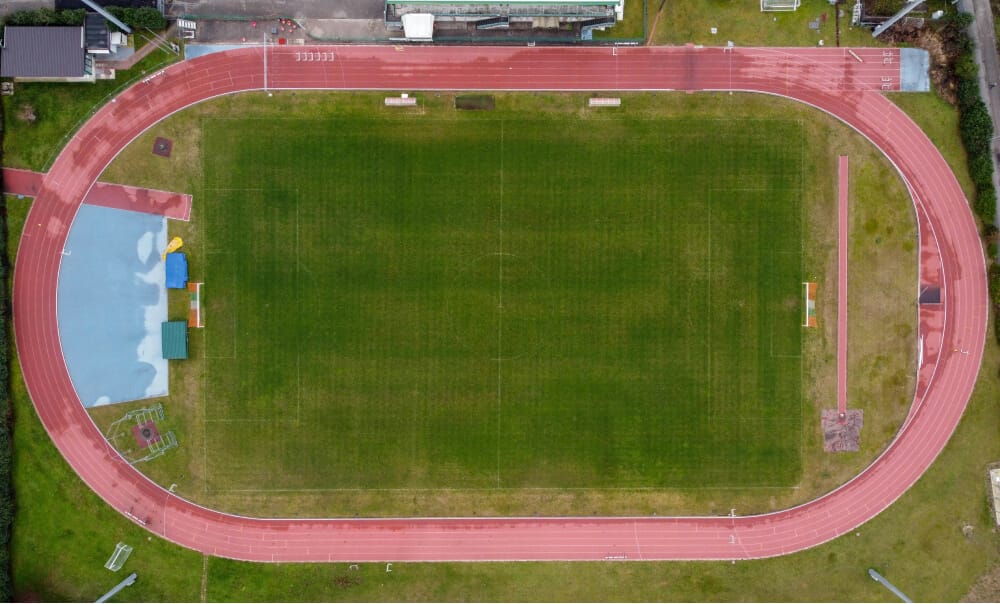
Just like a wild open running track that covers 10000 meters, a stadium running track will still help you conduct a 10000-meter race. But the runners will have to run 10 laps.
But unlike a wild open running track, A stadium running track doesn't occupy much space and is easier to maintain with little risk.
Anyway, in the next lesson, we will write our first loop.