Scenario 2: Get form details after the form submission
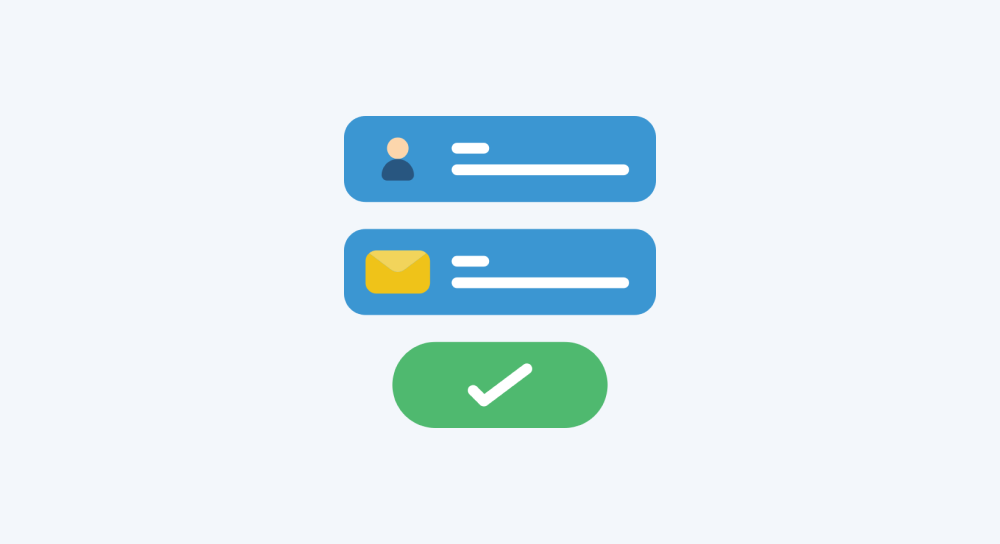
This is a common but lazy scenario where we provide feedback after the entire form is submitted.
This scenario can be achieved in four easy steps:
- Attach the
submit
event listener to the entireform
element instead of individual fields inside it - Once the form is submitted, select individual fields manually using the
document.querySelector()
method - Access the values of individual fields one by one
- Process the field values to validate the submitted form and finally provide feedback
Step 1: Attach the submit
event listener to the entire form
element instead of individual fields inside it
And prevent the default submission of the form to avoid page refresh.
You can achieve this by calling the event.preventDefault()
method immediately after the form submission event is fired.
const accountForm = document.querySelector(".account-form");
accountForm.addEventListener("submit", function (event) {
event.preventDefault();
//Access individual form field's value and process it
});
If we don't stop the default behavior of the form submission, then we can not access the form field data because the page refresh wipes the data out.
Step 2: Once the form is submitted, select individual fields manually using the document.querySelector()
method
We can do this inside the event handler after the submit
event is fired.
const accountForm = document.querySelector(".account-form");
accountForm.addEventListener("submit", function (event) {
// Prevents the default form submission behavior
event.preventDefault();
//Select all the fields individually so that you can access their values
const fullNameField = document.querySelector("#full-name");
const emailField = document.querySelector("#email");
const ageField = document.querySelector("#age");
const countryField = document.querySelector("#country");
const passwordField = document.querySelector("#password");
});
Because we are not trying to provide immediate feedback to the user, it's okay if we manually select each form field using the document.querySelector()
method and get its value after the form submission.
Also, in this scenario, we can not use event.target
or the this
keyword for selecting the individual form fields and getting their values.
This is because both event.target
and the this
keyword represent the main form
the element inside the event handler.
event.target
and this
keyword represents the HTML element on which the event is generated. Step 3: Access the values of individual fields one by one
const accountForm = document.querySelector(".account-form");
accountForm.addEventListener("submit", function (event) {
// Prevents the default form submission behavior
event.preventDefault();
//Select all the fields individually so that you can access their values
const fullNameField = document.querySelector("#full-name");
const emailField = document.querySelector("#email");
const ageField = document.querySelector("#age");
const countryField = document.querySelector("#country");
const passwordField = document.querySelector("#password");
//Get the value of "Full Name" field.
console.log(fullNameField.value);
//Get the value of "Email" field.
console.log(emailField.value);
//Get the value of "Age" field.
console.log(ageField.value);
//Get the value of "Country" field.
console.log(countryField.value);
//Get the value of "Password" field.
console.log(passwordField.value);
});
Now, because we selected the individual form fields using the document.querySelector()
method, we got access to those fields in the form of a Javascript object, right?
So, we can directly access the value
property on those objects to access the values entered by the user for each field.
Step 4: Process the field values to validate the submitted form and finally provide feedback
Now that we have access to all the form data entered by the user, we can process the data and provide feedback.
We can't do this right now because we didn't learn about advanced concepts yet.
There are other advanced ways to access the form data all at once
For example, by calling the FormData()
constructor function after the form submission, we can get access to all the form data as a unified data set.
const accountForm = document.querySelector(".account-form");
accountForm.addEventListener("submit", function(event) {
// Prevents the default form submission behavior
event.preventDefault();
// Create a new FormData object from the form element
const formData = new FormData(accountForm);
// Access form data directly using spread syntax
const formDataEntries = [...formData.entries()];
// Process each entry (field name and value)
formDataEntries.forEach(([fieldName, fieldValue]) => {
console.log(`Field Name: ${fieldName}, Field Value: ${fieldValue}`);
// You can now perform validation and processing on each field value here
});
});
After the form submission, executing the above code would result in the following data in the console:
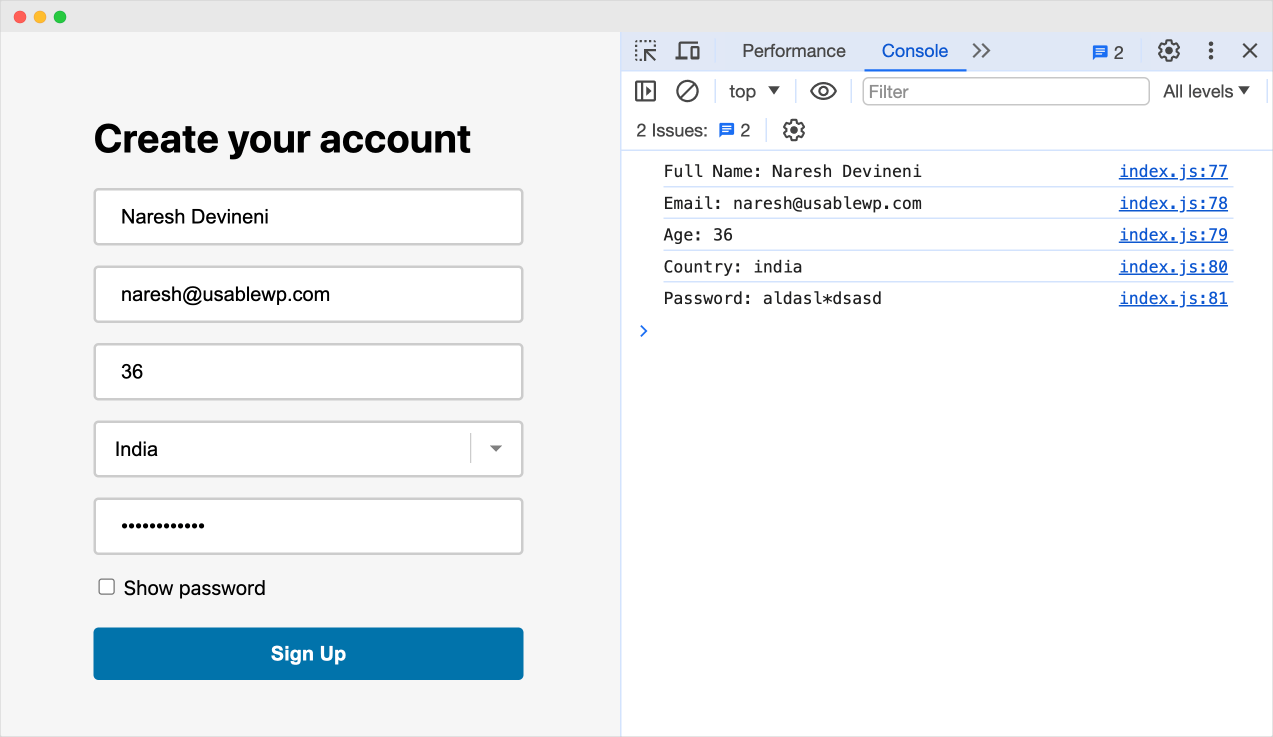
But if you want to use the FormData()
function, you need to understand the concept of "Constructors" and "Loops" in Javascript.
We will cover both of those concepts in the upcoming modules and then learn how to use them with forms.
For now, let's just get good with the scenarios explained during this lesson and the previous lesson.
In the next lesson, we will learn how to improve the performance of the final code for this lesson.