Javascript Variables
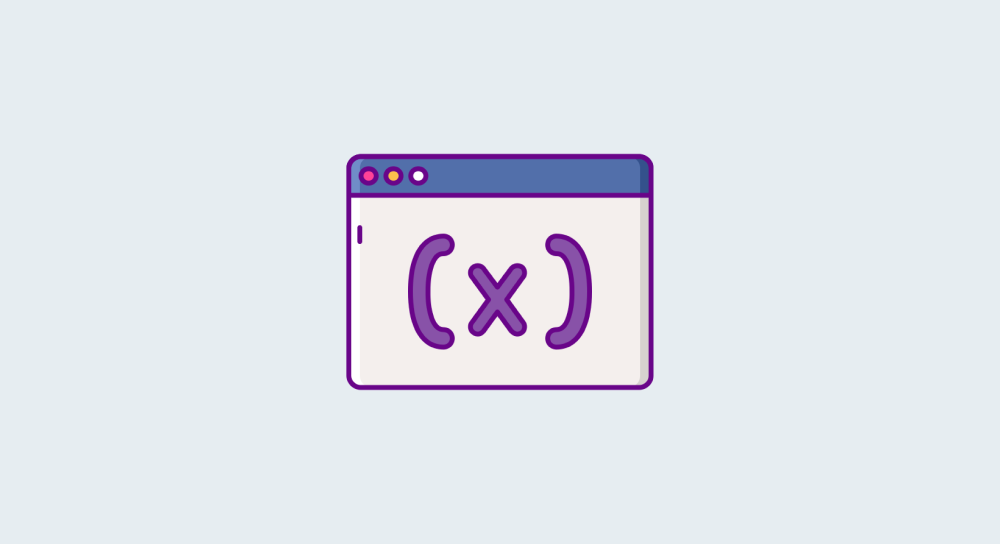
In the last few lessons, we learned how to collect data from a visitor and then how to access it.
But all the information we were collecting is going to waste because we are not saving it anywhere.
It is just like forgetting to put a cup to hold the coffee that is being dispensed by the vending machine.
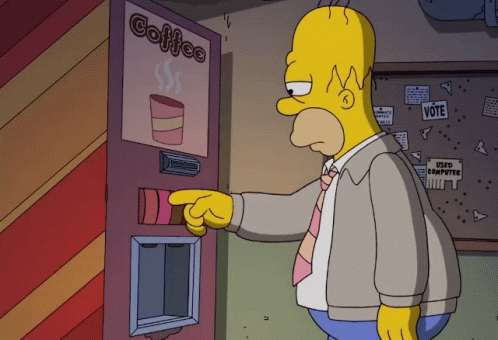
So, how do we fix this situation?
Simple. We have to use Variables to save the data collected.
So, what are variables?
In programming, Variables help us store a piece of data so that we can retrieve it at a later point in time.
And we usually retrieve that data to:
- Display it
- Pass it around
- Manipulate it.
This list goes on and on...
Variables are similar to a cup that holds the coffee.
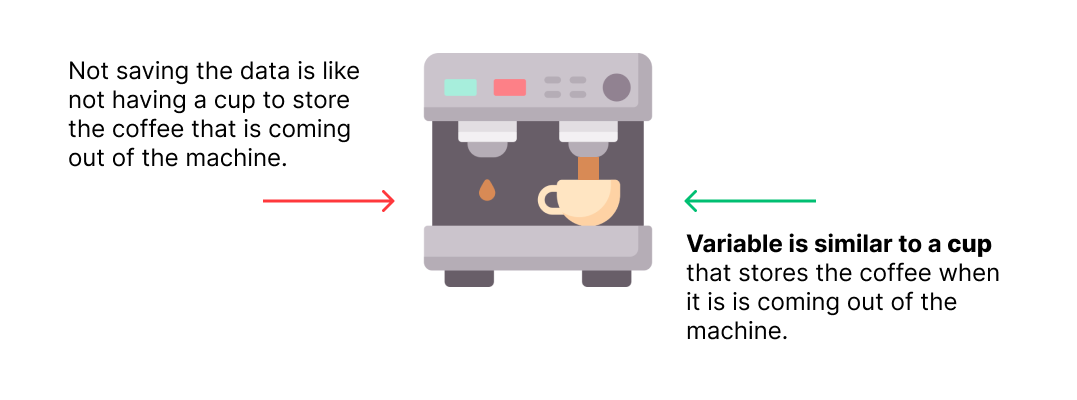
Actually, we can easily understand the usage of variables using the box analogy instead of the coffee cup analogy.
Imagine a variable as a box with a unique label and we can store data inside that box.
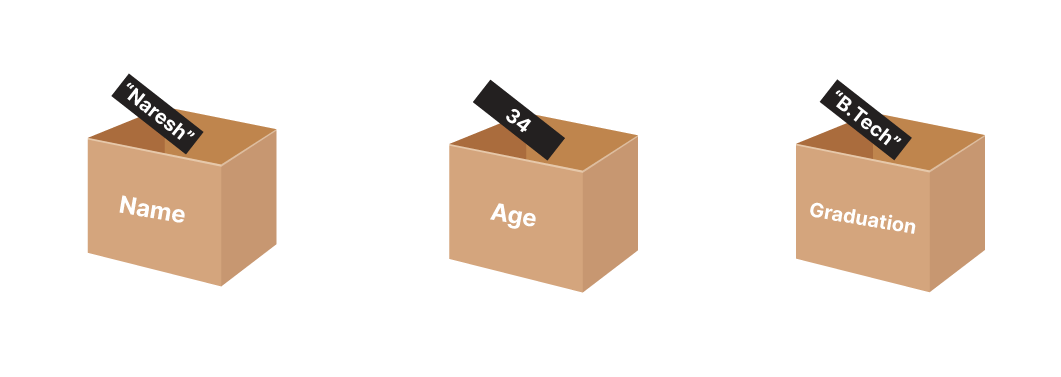
The data can be of any type. Javascript supports a lot of data types such as text, numbers, etc. and we will learn about them shortly after this lesson.
We gave each box a unique label because we will use that label to retrieve the data from that particular box at a later point in time.
We can also update a variable any number of times
And, whenever we update a variable with new data, the previously stored data will be deleted and can no longer be accessible.
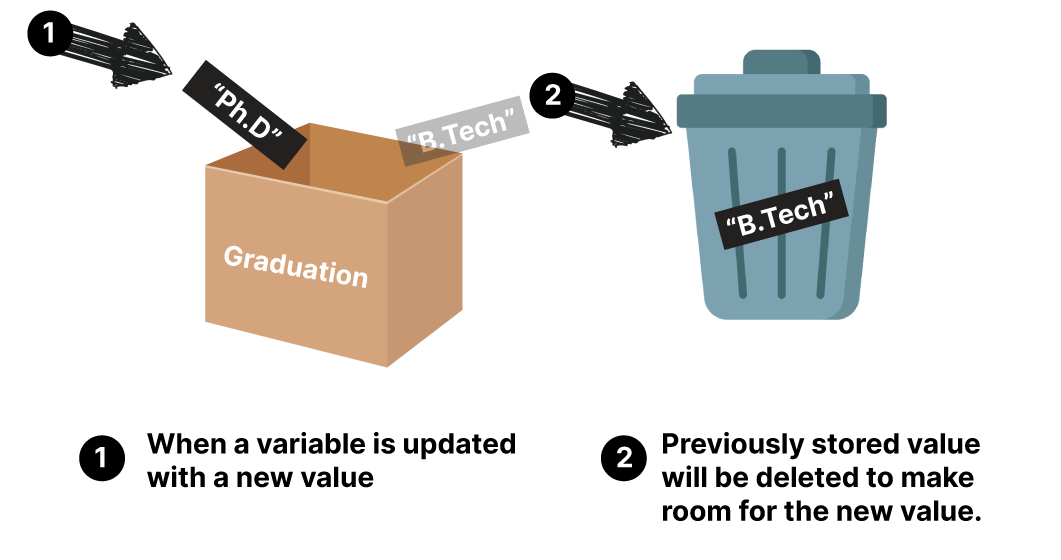
After all, the word "variable" means something that might change, right?
Anyway, enough theory!
How do we create a variable and save data in it?
We can do that in two easy steps:
//Step 1: Declare a variable by using the keyword "let"
let age;
//Step 2: Use the name of the variable to assign a value to it
age = 34;
Step 1: Declare a variable by giving it a name
The terminology for creating a variable is called declaring a variable or variable declaration.
And, we have to use the keyword let
to declare a variable:
let age;
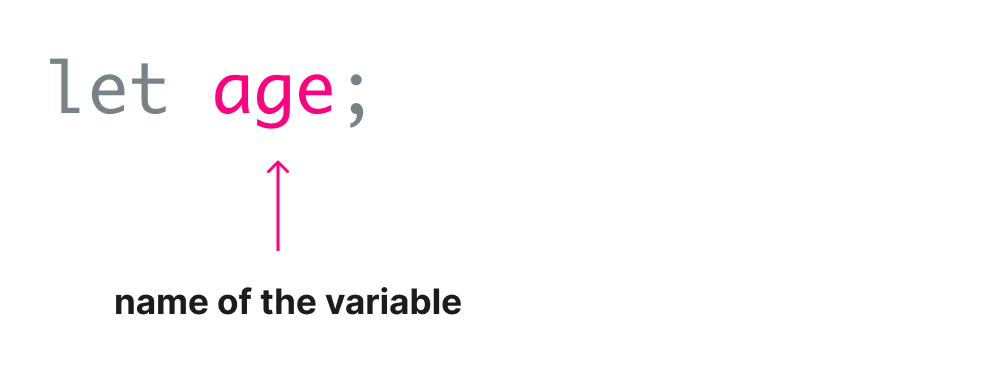
And we have to name it because we have to use that name later.
This will tell the computer that "Hey! Create a memory location for the variable named age
so that I can store some data in it."
In the terminology of Javascript, a variable name is called Identifier
.
You can name the variable anything you want. There are some rules for naming variables and we will take a look at them shortly.
Anyway, the sole purpose of declaring a variable is to store data in it, correct?
So, now comes the next step.
Step 2: Use the name of the variable to save data in it
Saving data inside the variable simply means assigning a value to it.
We can save data inside a variable by using its name and the =
( equal sign ).
For example:
age = 34;
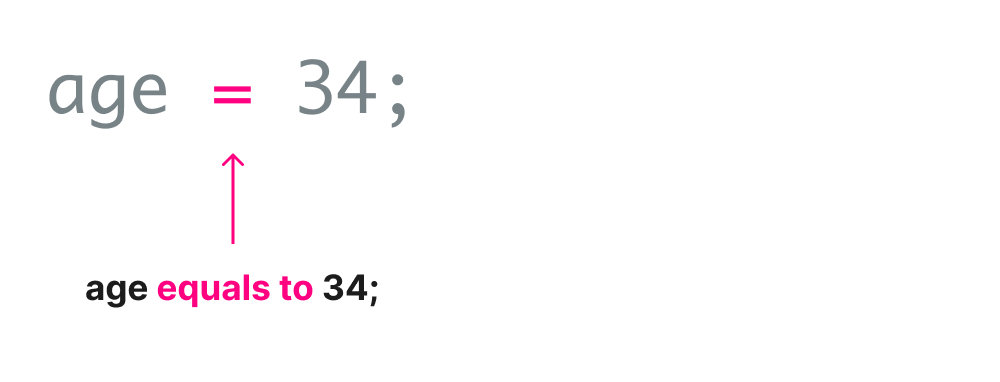
This will tell the computer that "Hey! go ahead and access the memory location called age
and store the value 34
in it."
In other words, the variable age
is used as a reference to access the value 34
.
Just to recap, if we want to create a variable and store some data in it, there are two steps involved:
//Step 1: Declaring a variable
let age;
//Step 2: Assigning data to that variable
age = 34;
Easy, right?
If you notice, we have manually created a data called 34
and stored it inside the age
variable.
Technically, we are hard-coding the data ourselves.
There is nothing wrong with this.
We will do this quite a lot while working on practical projects too.
But sometimes, we have to store some dynamic data as well.
For example, in the last lesson, we are collecting the age dynamically from the user using the prompt dialog box, right?
We call this dynamic data because we are getting data as a return value from a function execution and we are not hard-coding it.
So, how do we store that dynamic data in a variable?
The same rules apply here as well.
Come on, let's take the example of a prompt
function that asks the user for age:
prompt("What is your age?");
And, we already know that the execution of the above function is returning a dynamic value submitted by the user.
In this case, the dynamic value is age:
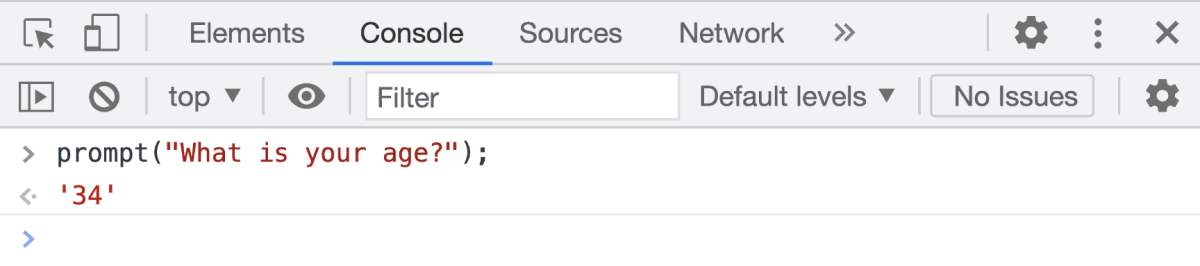
Now all we have to do is to assign that function
execution to a variable:
age = prompt("What is your age?");
And this will save whatever the data returned by the execution of the prompt
function inside the age
variable.
If we translate the above line of code to plain English, it sounds like:
The age
equals to "whatever the value entered by the user".
Basically, this will tell the computer that:
"Hey! go ahead and access the memory location called age
and store whatever the data returned by the execution of the prompt
function inside it."
That's it. Nothing complicated.
Simple enough, right?
Just to be clear, I will show you what's happening with the help of a Console.
And, I want you to repeat the same steps shown in the video on your console.
Did you do it?
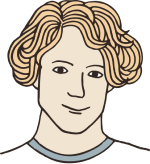
Yep!
Cool, in the next lesson, we will learn just a few details about Variables and then you are ready for your next simple exercise.