Javascript Syntax and Errors
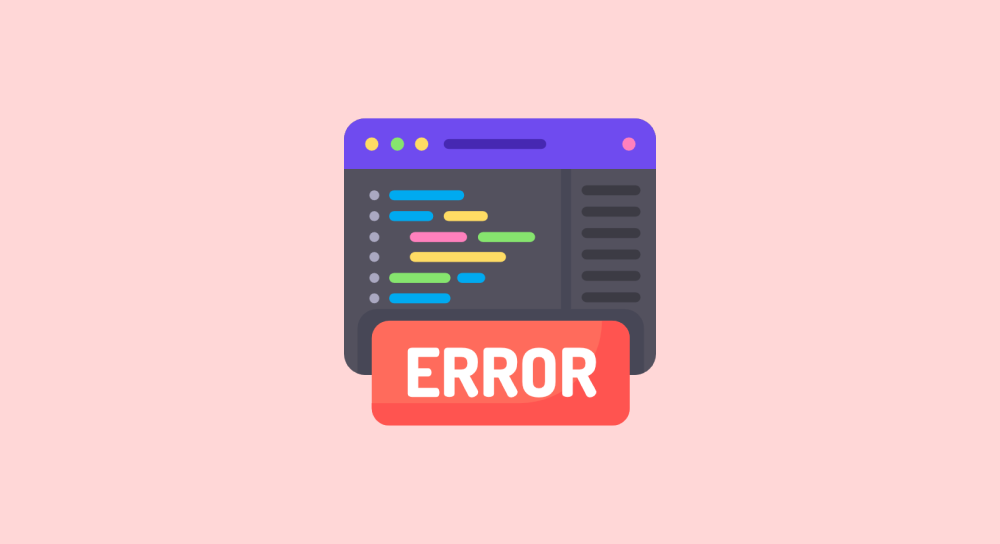
When we wrote our HTML and CSS code, even if we didn't follow any standards, we never used to see any errors in the browser.
That means, when it comes to HTML and CSS, the browser is a bit lenient and doesn't throw any errors.
But when it comes to Javascript, when you do not write code by following certain rules, the browser will yell at you by throwing an error.
Now don't get scared.
This is where Syntax comes in.
Whenever we are using a particular feature in Javascript, if we can read the syntax of that feature, we'll avoid errors completely.
So, what is syntax in programming?
A coffee vending machine's user manual will always contain a section dedicated to the precautions list, right?
If we don't follow those precautions, the coffee vending machine might get damaged.
Similarly, when you write in English, you follow the grammar rules of English so that meaning of what you write doesn't change with bad English.
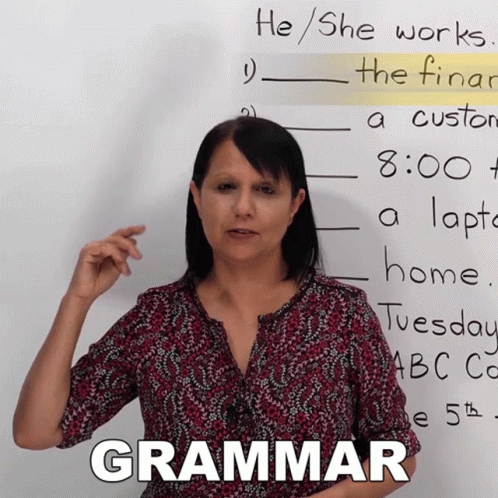
Similarly, the Syntax is nothing but a set of rules that we must follow when typing the code.
If we break the rules related to syntax, Javascript will throw errors and some of the interactivity on the page will come to a halt.
For example, here is the syntax of the alert
function:
alert(message);
What if we don't follow the syntax and put parentheses in front of the function name instead of next to it?
(message)alert;
Come on, go ahead and type the following code inside the browser console:
("Do you think this code works?")alert;

And bang! Syntax Error!
Javascript engine threw syntax error because it doesn't recognize the above line of code as valid syntax. And it doesn't understand that code either.
One more example. What if we don't use parentheses at all?

This time, we kept the order intact but we have removed the parentheses.
But Javascript still threw an error.
The thing is, the Javascript engine follows a certain rule book when executing any line of Javascript code and if our code deviates from those rules even slightly, Javascript will throw an error.
It means that we should always follow the syntax.
No ifs. No buts.
No bargaining.
And this is why when you are trying to code Javascript, always watch out for errors and especially syntax errors.
For example, let's take a look at the alert
syntax again.
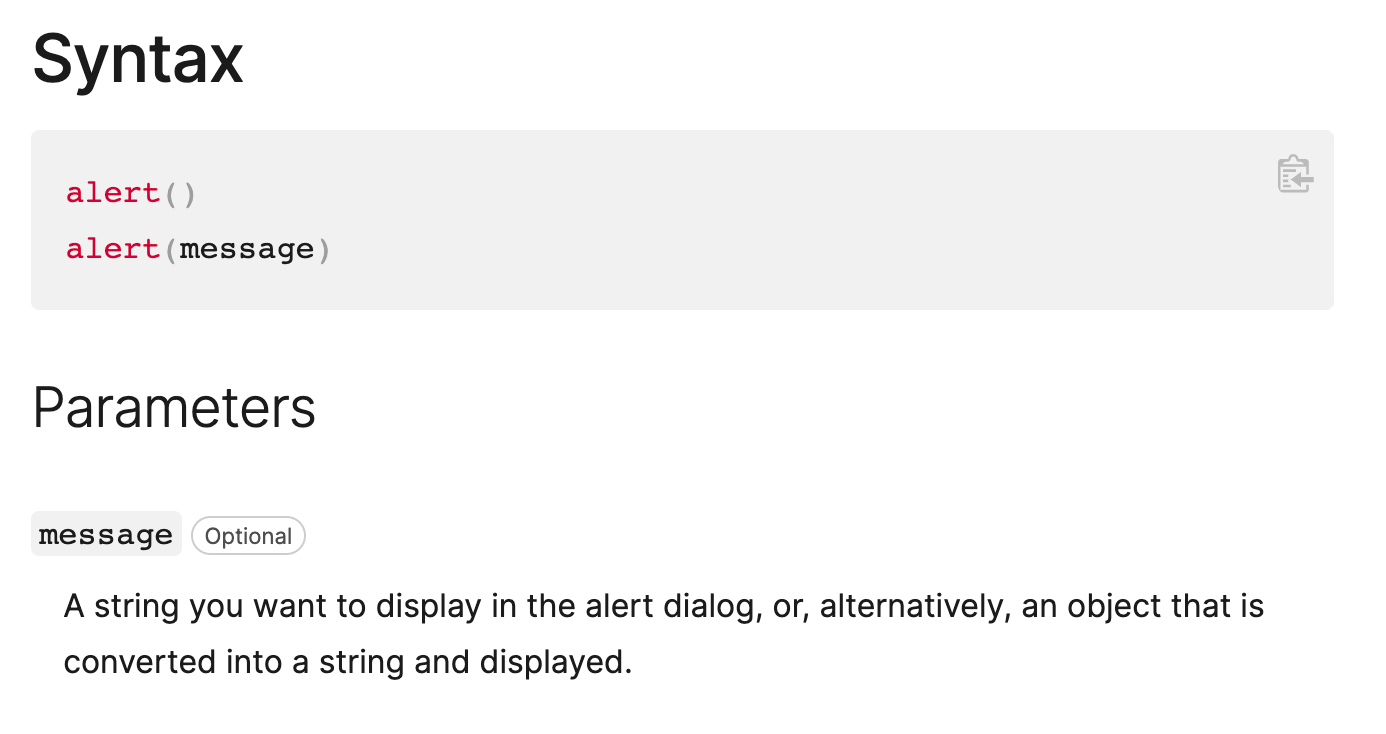
Based on the syntax, The only way to use the alert
function is:
//Parenthese must come after the name of function
alert();
// If we are providing a message as an input, The message must be surrounded with quotes
alert("This code works");
But just in case you forget the quotes, the Javascript will throw an error:

You get the idea, right?
And the thing is, Syntax Error is only one type of Javascript error. There are a lot of other types of Javascript errors.
We will look into them as we progress through the course.
Long theory short, we can avoid errors by reading the "Syntax" section of MDN documentation.
Javascript errors are destructive
The problem with Javascript errors is that they will stop the web page from functioning properly.
It is better shown than explained and I will practically show this when the time comes.
For now, in the next lesson, we will put will all our knowledge about Functions together to build a very small project.