Javascript Functions 101
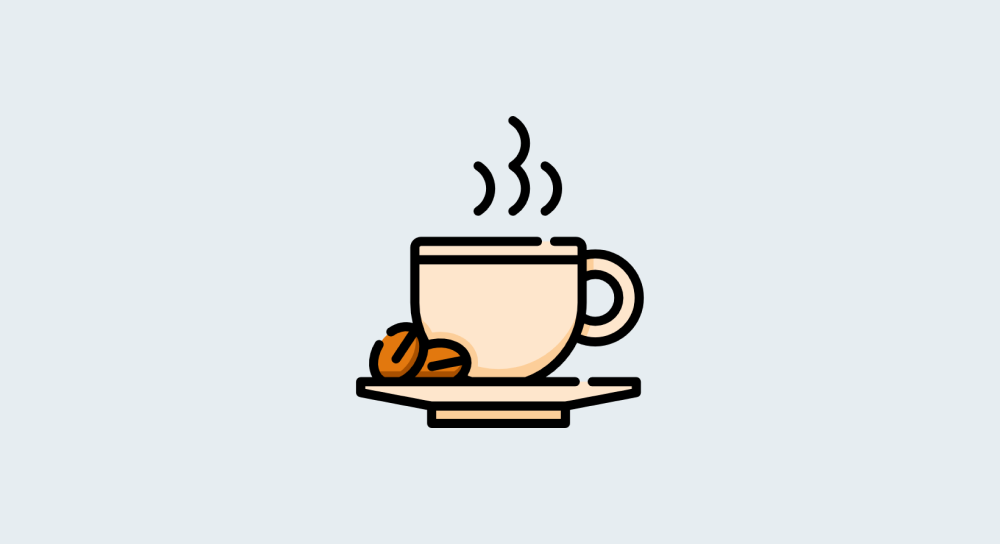
Have you ever come across a simple coffee vending machine?
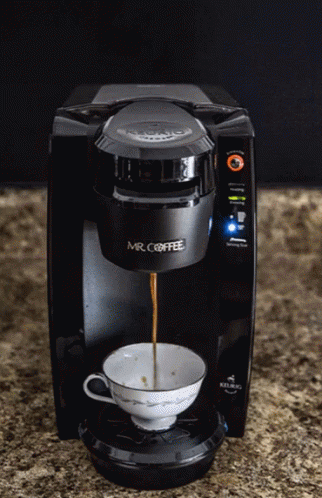
A coffee vending machine has only one job, and that is to make a fresh brew of coffee.
It doesn't do anything else. It only performs one task. Making coffee.
You don't have to boil the water by yourself. You don't have to heat the milk. You don't have to crush the coffee beans by yourself.
A coffee vending machine will automatically perform all the steps for you, saving you a lot of time.
You only have to push the button to trigger the coffee machine to make a coffee for you.
Easy, right?
In the programming world, a function
is just like a coffee vending machine.
A function
is used to perform a particular task over and over again.
Most of your work as a programmer revolves around using many in-built functions, or you'll create custom functions to get individual tasks done.
So, let's spend some good time understanding the basic characteristics of a function
in Javascript.
And I will provide enough examples to make sense of each characteristic.
Ideally, a Function is responsible for performing one task and one task only
Just like a coffee machine, a function
in Javascript is created to perform a single task.
For example:
- Showing a popup when a button is clicked
- Increasing the font size of the web page when a button is clicked
- Changing the slider image when the "Next" or "Previous" button is clicked
- Getting the current year to show it in the footer
- Getting the current weather information in NewYork
- Removing an item from the shopping cart
If you notice, each task is focused on doing only one job, such as showing a popup, increasing the font size, etc.
We will work on all these tasks as we progress through this course.
For now, the best example I could give is Javascript's alert
function.
Yes, the alert()
that we used in the last exercise is an in-built function
in Javascript.
If you think of it now, the only task of the alert
Function is to alert the user with a message.

Similarly, the only task of the prompt
Function is to ask a question to the user and get an answer to the question.

Finally, the only task of the print
Function is to open up the print dialog box of the browser:
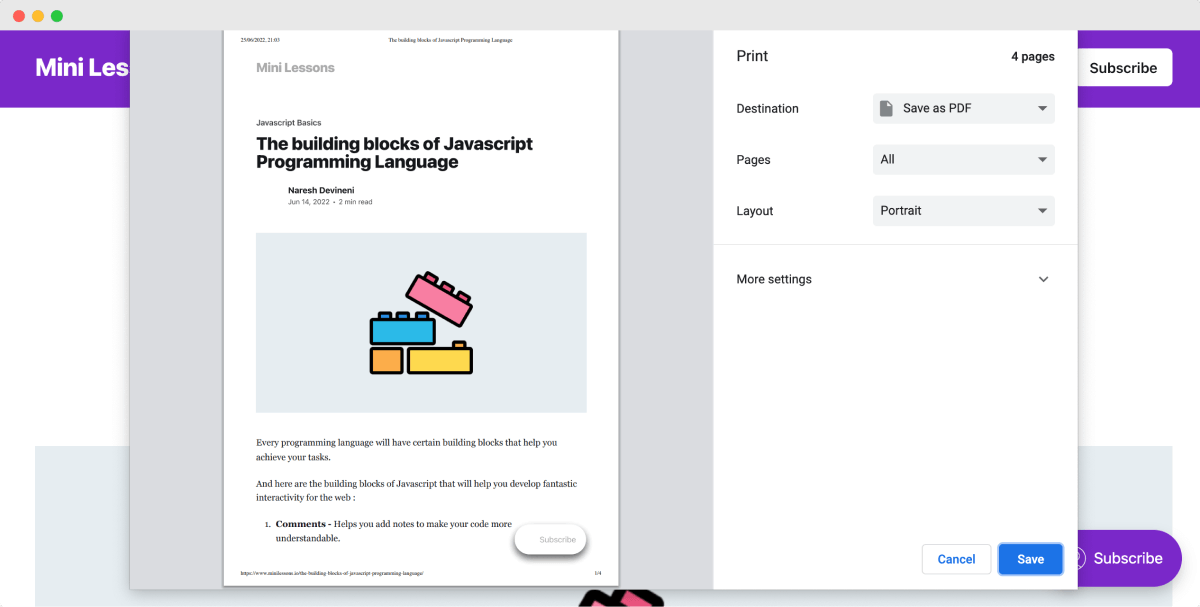
A Function has a name so that we can use it
For example, alert
is the name of a function.
Similarly, print
and prompt
are the names of a function.
As you can see, the function names are self-descriptive too.
The function name alert
tells us it is related to alerting the user about something.
Similarly, prompt
tells us that it is related to prompting the user for something.
You get the idea, right?
A Function can be triggered to make use of it
A coffee machine doesn't dispense the coffee on its own.
You have to instruct it somehow, saying, "Hey! Give me a cup of coffee."
And in most cases, unless there is some automated schedule involved, you have to push a button on the machine for it to start making coffee for you.
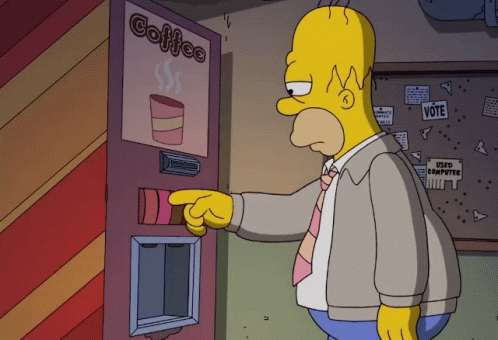
You pushing the button is one of the triggers for the coffee machine to produce a cup of coffee.
Similarly, a function
in Javascript won't get triggered automatically.
You have to trigger it, and one way to do that is by putting the parentheses ()
next to its name.
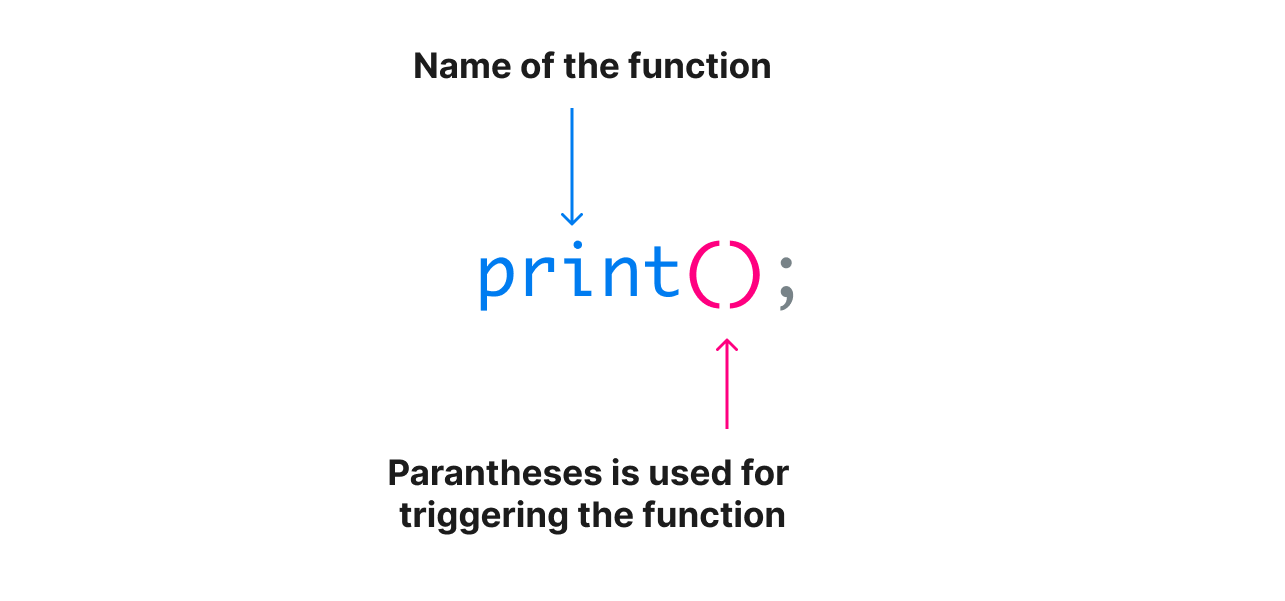
In other words, the parentheses ()
act as the push button.
For example: alert();
or
print();
In the terminology of programming, we call this Function triggering as:
Executing the Function or Calling the Function.
This means that If you try to call alert
function without the parentheses ( )
, the browser does nothing.
alert;
This will do nothing!
It doesn't trigger the popup.
So, the parentheses are important to bring a Function
to life.
Anyway, sometimes, a function
needs more than just ()
to be useful.
Inputs to be more precise.
We will learn about them in the next lesson.