How to access the items inside an array
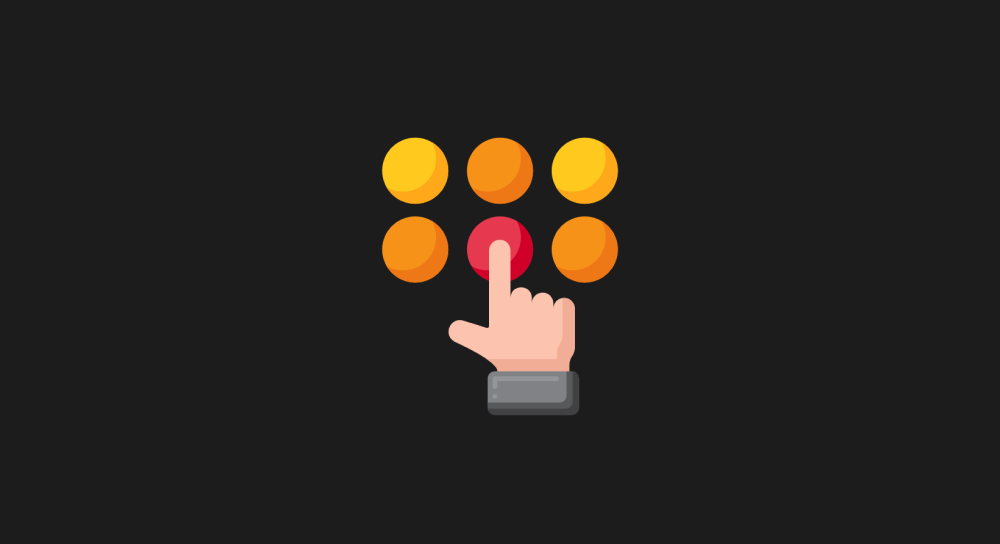
You are storing a collection of data inside an array for a reason.
You want to access that data at some point and do something with it.
Anyway, you can access the data of an array the same way you access normal variables.
As you already know, If we want to access the value we stored inside a variable, we have to use the variable's name.
For example, let's just say we have a variable called hobby
, and it has the value "Playing Cricket"
stored in it.
let hobby = "Playing Cricket";
We can access the value "Playing Cricket"
by using the variable name hobby
like this:
hobby;
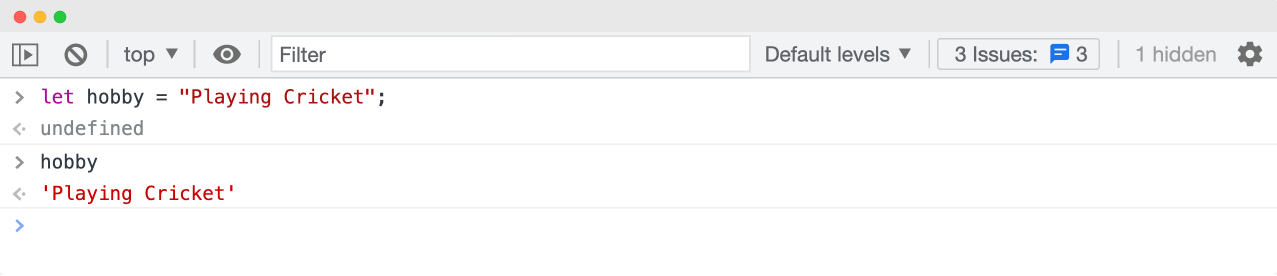
Similarly, If we want to access the array of data stored inside a variable, we must use the variable's name.
Come on, let's try it out.
Open up the Browser Console and type the following code:
let iPhones = ["iPhone 13", "iPhone 12", "iPhone 11"];
We have created an array called iPhones
, and we have stored three items in it.
Next, let's go ahead and access the iPhones
variable and see what happens:
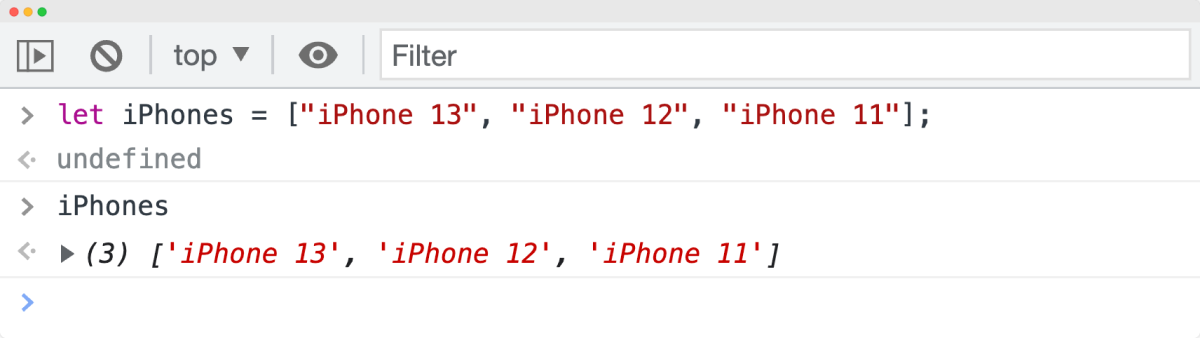
As you can see, we can now access all the items stored as an array.
This is good.
But what if you want to access just one item from an array?
Accessing only a single item from an array is a pretty common scenario.
How to access a single item inside an array
For example, let's say you only want to access the third item inside iPhones
array.
How do you do it?
This is where something called the array item's index comes in.
Introducing Array item index
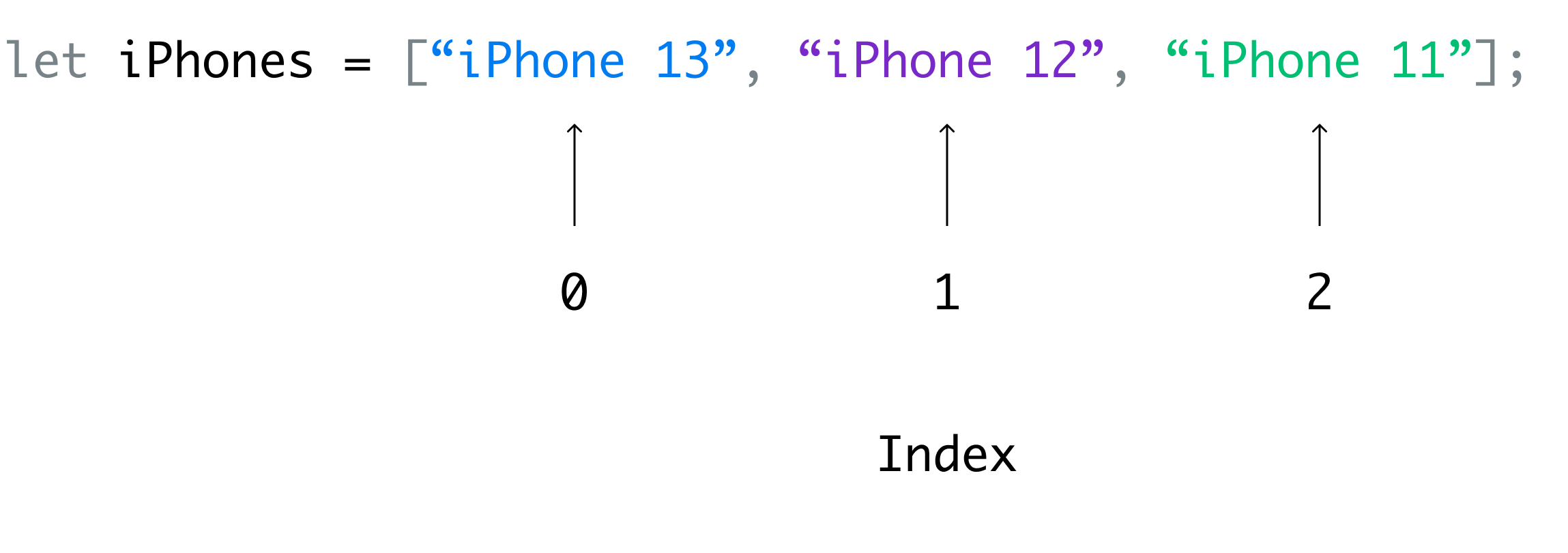
The thing is, each item stored in an array is given a unique index
automatically by Javascript.
An index
is a number, and it starts from a zero.
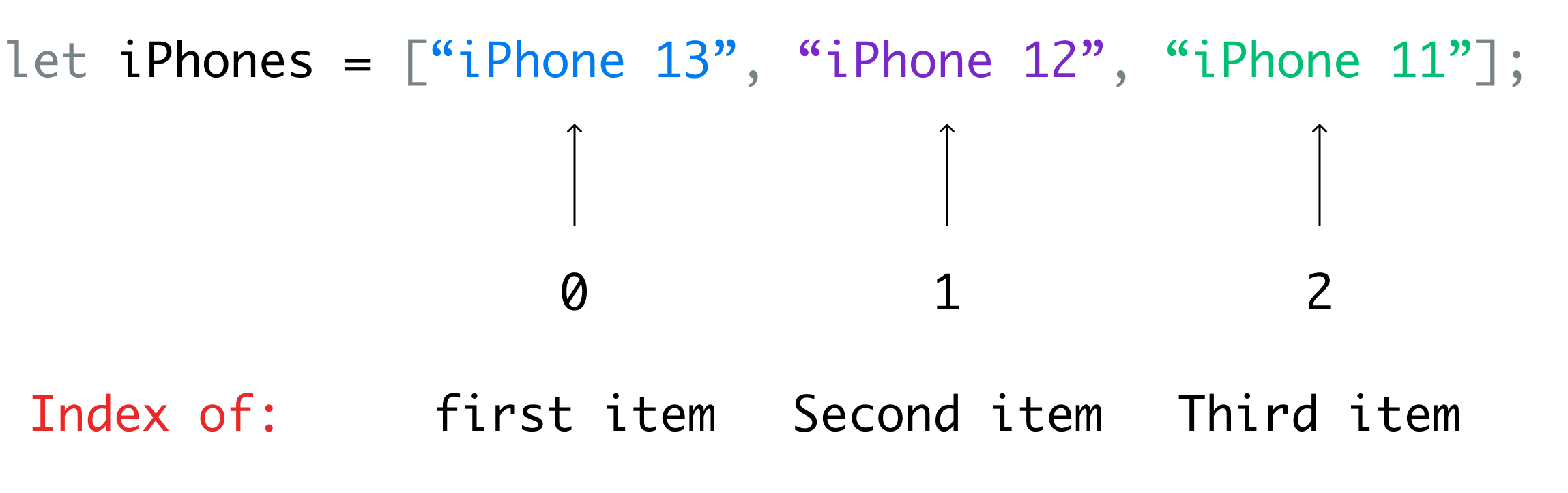
For example, the index
of the first item inside the iPhones
array is 0
.
Similarly, the index
of the second item 1
.
The index
of the third item 2
.
And if you notice, the index
number will keep incrementing based on the length of the array.
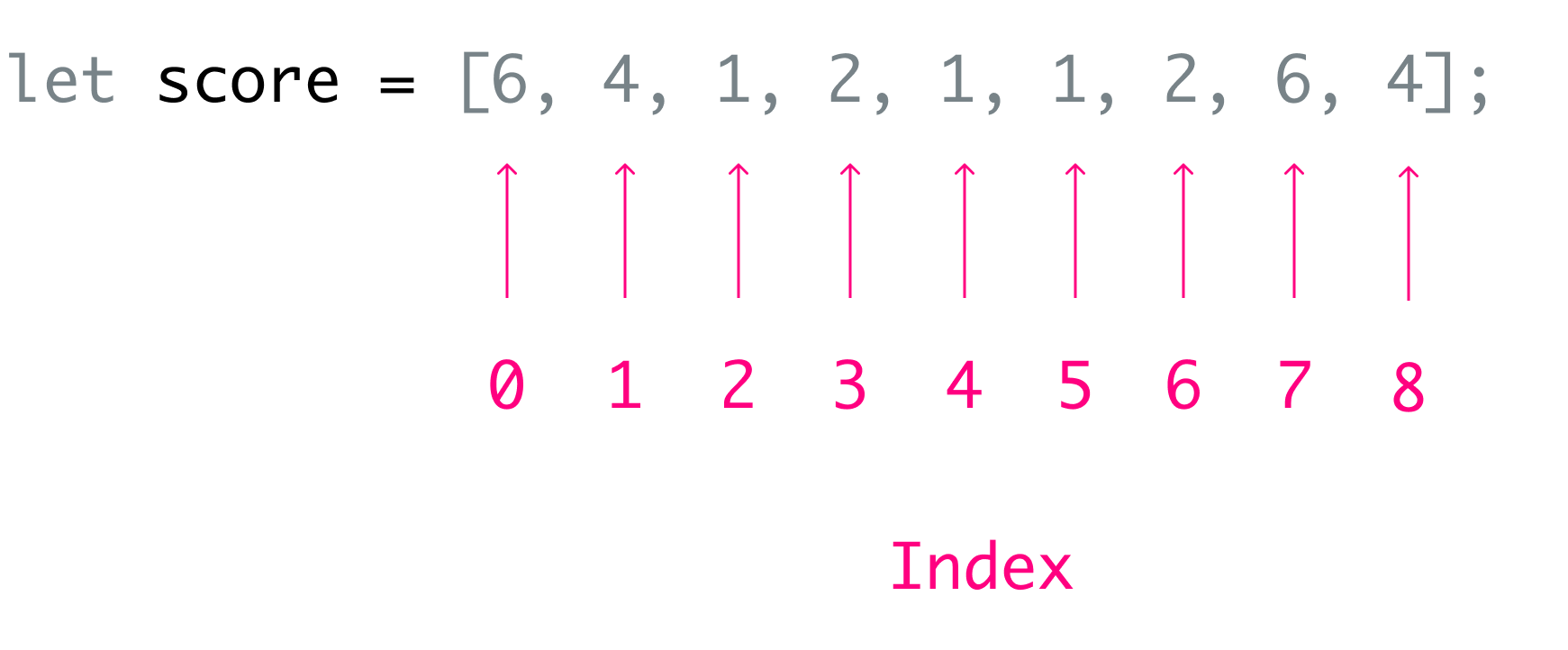
For example, if an array has nine items inside it, the index of the last item is 8.
As simple as that.
This concept applies to any array that you create inside Javascript.
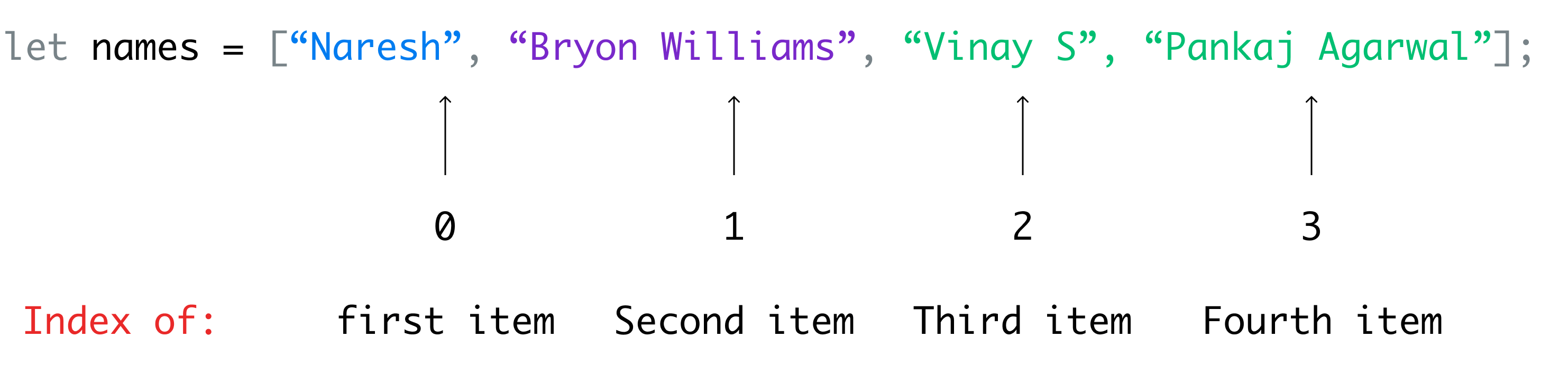
And I can't stress it enough.
The index
of the first item inside any array is always 0
.
Similarly, the index
of the second item inside any array is always 1
.
You get the idea, right?
Anyway, now that we know that each item has an index, to access a particular item inside an array, all we have to do is provide the item's index number in square brackets:
arrayName[index];
We must use the name of an array and then provide the item's index inside the square brackets.
For example, let's go back to our iPhones
array:
let iPhones = [“iPhone 13”, “iPhone 12”, “iPhone 11”];
Now, I want to access the third item "iPhone 11"
:
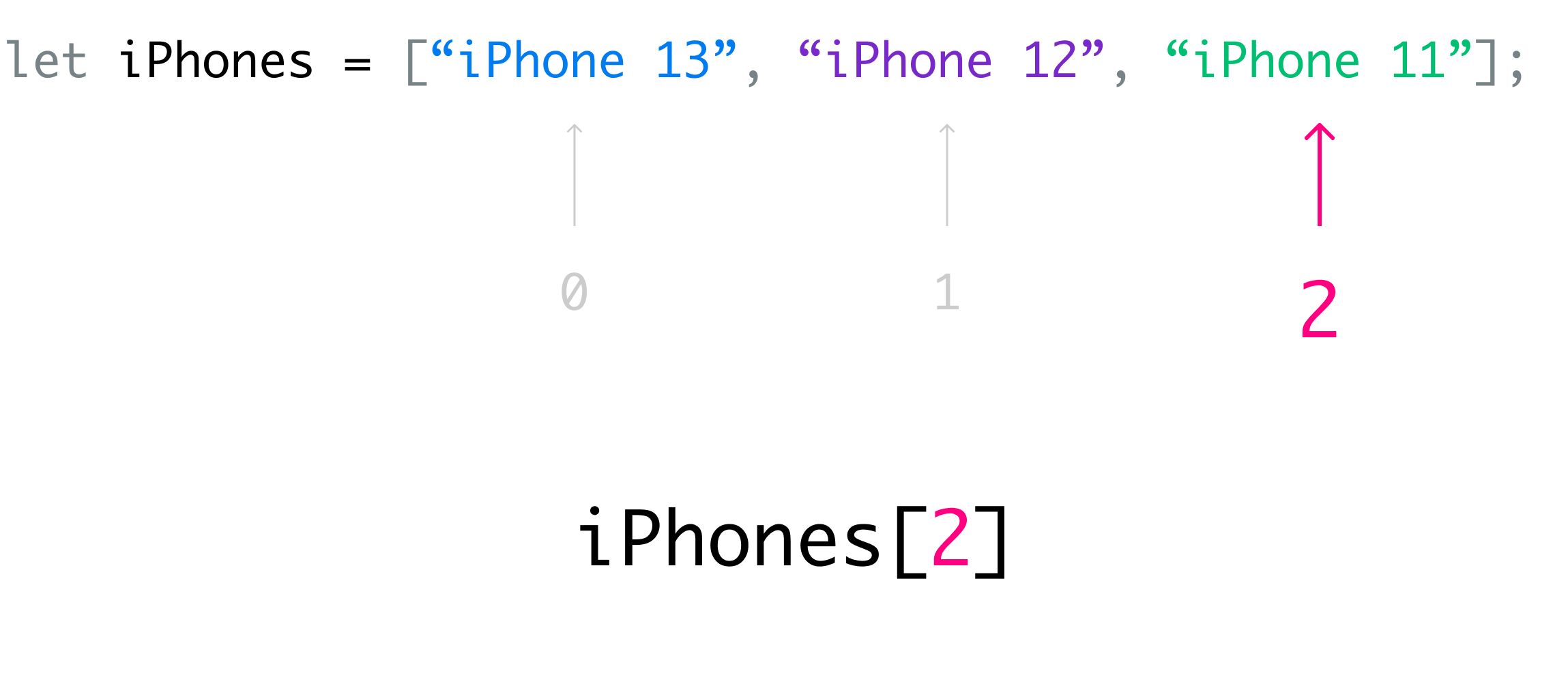
If you start counting from 0
, the index of the third item is 2
, right?
So, we can access the item "iPhone 11" by providing 2
as the index value:
iPhones[2];
// Returns "iPhone 11"
Another quick example: Let's say there is a names
array and it has four items stored in it.
let names = [“Naresh”, “Bryon Williams”, “Vinay S”, “Pankaj Agarwal”];
If you want to access the second name "Bryon Williams"
, here is how you do it:
names[1];
// Returns "Bryon Williams"
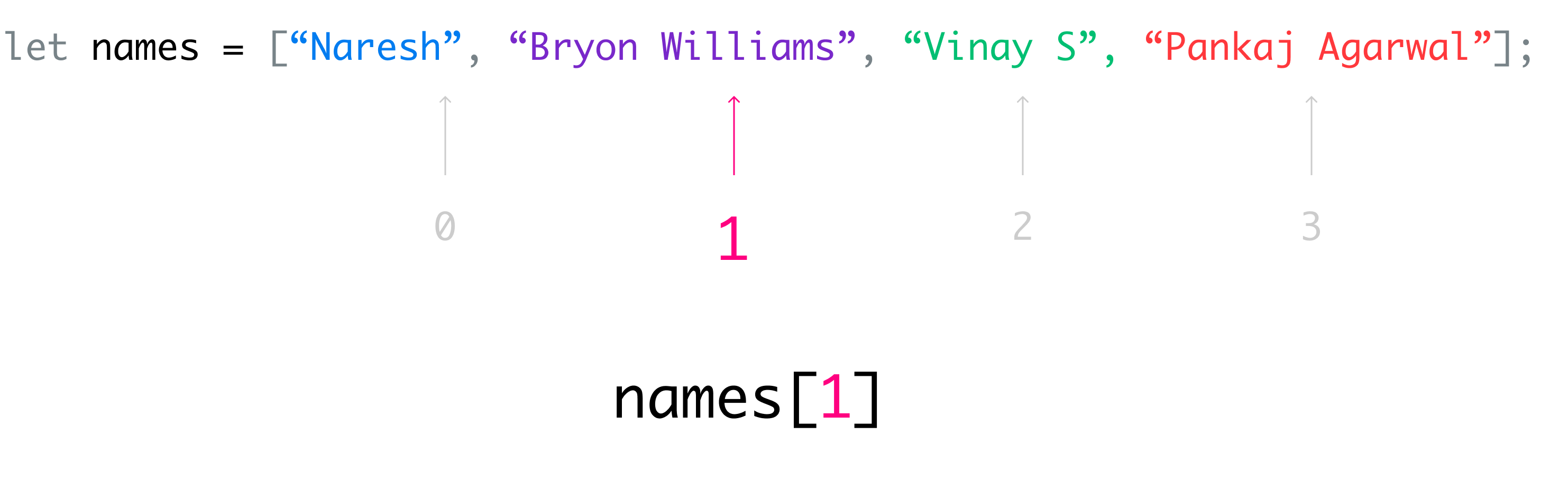
Easy enough?
Now that you understand how to access items inside an array, let's perform some small exercises starting from the next lesson.