Data types: undefined and null
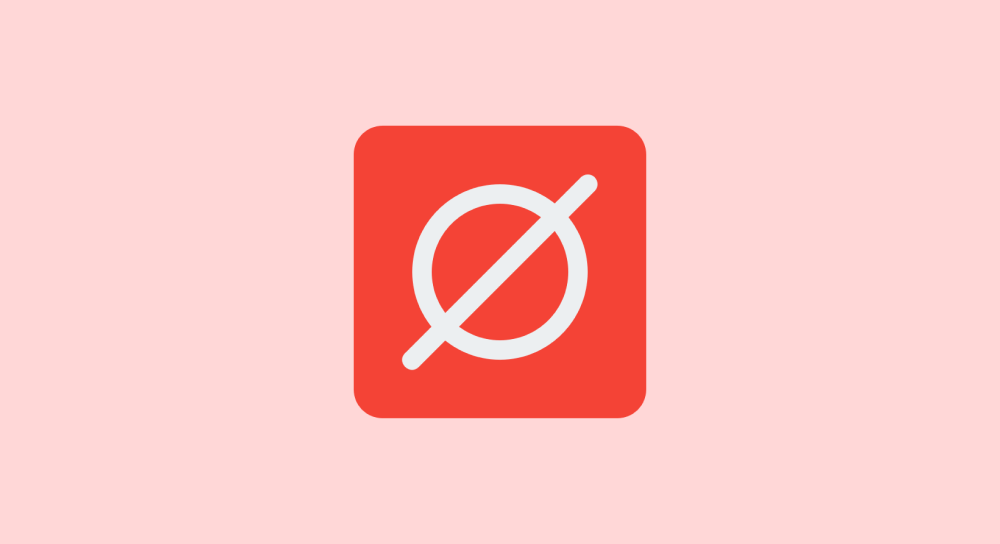
Yes, both undefined
and null
can be used as values in Javascript.
You'll come across a lot of undefined
and null
values when you are creating components. So, it is important you know the difference between them.
Most people think that both null
and undefined
values are the same.
Agreed. They both can be used when there is a need to store an empty value inside a variable. No one will police you.
But when it comes to the internals of the Javascript programming language, they are different, and they have different purposes.
To understand how they are different, Open up the Console. It's important for this lesson.
undefined
Whenever you declare a variable in Javascript, initially, a value of undefined
is assigned automatically to that variable.
To understand this, use the Console to declare a variable but don't assign any value to it:
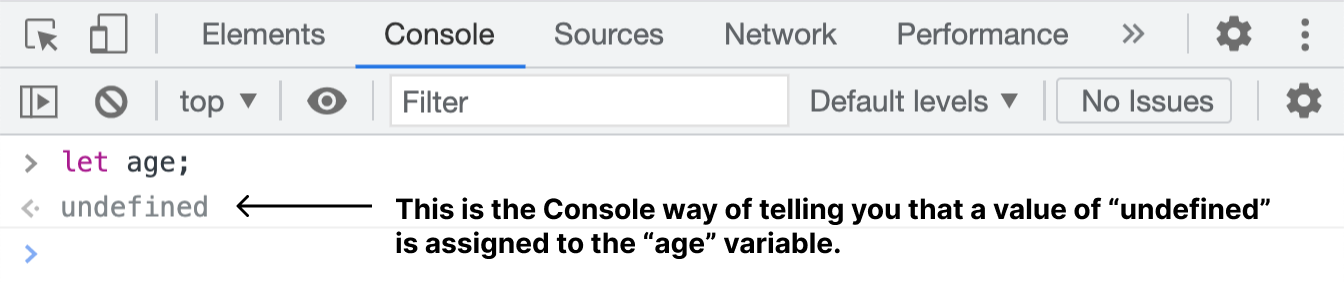
As you can see, first, we declared a variable called age
, but we didn't store any data in it.
As a result, instead of keeping the age
variable empty, Javascript stores the value undefined
in it.
That's all.
The undefined
value is the Javascript way of saying that:
"Hey! You declared a variable but did not store any value in it. So I am storing a using default value called undefined
for now. You can change this later".
This is the primary purpose of the value undefined
in Javascript.
There are some other important use cases, but we will talk about them later.
null
The null
value can be assigned to a variable.
let age = null;
It can be used when a particular piece of data is missing or not acquired.
In other words, it is intentional and not by accident.
To understand it, open up the console and use the prompt
function to ask a question to the user:
prompt("Are you married?");
This will bring a dialog box.
Now, instead of entering your marital status, hit the cancel button and notice the return value in the Console.
Did you see that?
The Console says that the prompt
function returned null
because you, as the user, canceled the dialog box by not answering the question asked.
Returning null
is the Javascript way of saying that:
"Hey! The user did not enter any value. So I am returning null
to you. So, when you see the null
as a return value, please understand that your question went unanswered!".
You can also say that null
value is similar to the "Not Applicable" option when asking someone to complete a form.
In Javascript, you'll usually find the null
value when dealing with HTML forms.
In the next lesson, we will talk about the most important data type called Objects.