Challenge: Outputting all the items of an array into the web page using a for loop
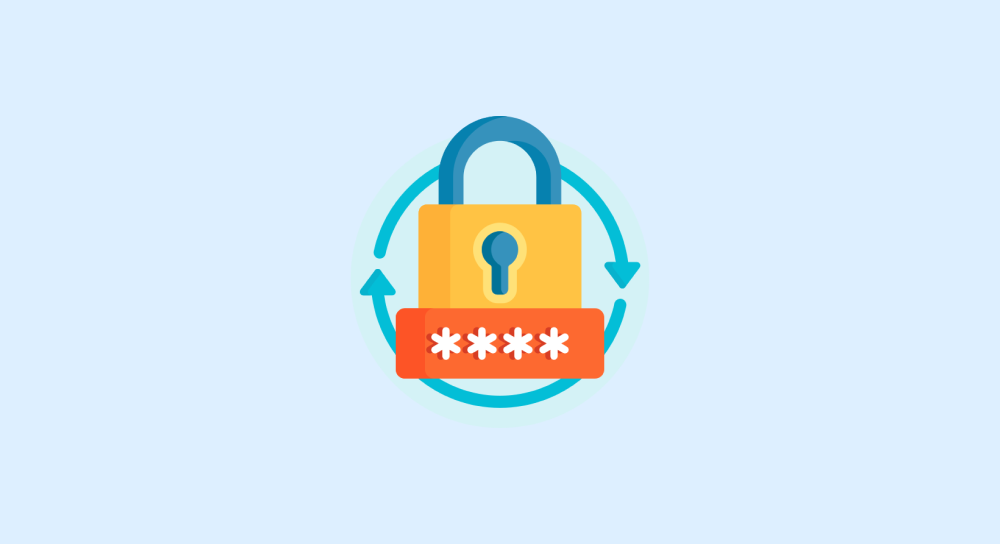
If you remember, we have previously worked on an exercise of outputting a list of unsafe passwords onto a web page:
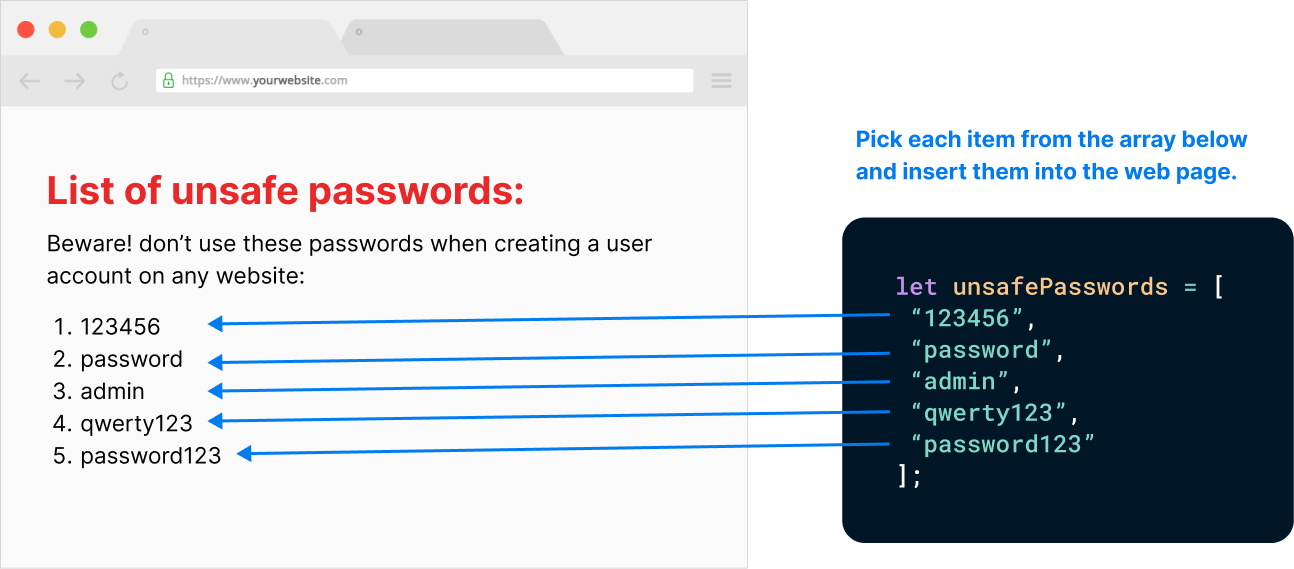
Here is the final Javascript code for that exercise:
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
//Select the parent element
const olElement = document.querySelector("#unsafe-passwords");
// Outputting the first item in to the web page
const firstLiElement = document.createElement("li");
firstLiElement.textContent = unsafePasswords[0];
olElement.appendChild(firstLiElement);
// Outputting the second item in to the web page
const secondLiElement = document.createElement("li");
secondLiElement.textContent = unsafePasswords[1];
olElement.appendChild(secondLiElement);
// Outputting the third item in to the web page
const thirdLiElement = document.createElement("li");
thirdLiElement.textContent = unsafePasswords[2];
olElement.appendChild(thirdLiElement);
// Outputting the fourth item in to the web page
const fourthLiElement = document.createElement("li");
fourthLiElement.textContent = unsafePasswords[3];
olElement.appendChild(fourthLiElement);
// Outputting the fifth item in to the web page
const fifthLiElement = document.createElement("li");
fifthLiElement.textContent = unsafePasswords[4];
olElement.appendChild(fifthLiElement);
If you remember, we were accessing and outputting each item from the array manually because we didn't know how to use loops back then
But now, we do know how to use a "for" loop.
So, here is a simple challenge that you already know how to solve:
First, download the project files for this challenge and open them inside your code editor:
Next, if you open up the index.js
file, it contains an array with a lot of items in it:
let unsafePasswords = [
"123456",
"123456789",
"12345",
"qwerty",
"password",
"12345678",
"111111",
"123123",
"1234567890",
"1234567",
"qwerty123",
"000000",
"1q2w3e",
"aa12345678",
"abc123",
"password1",
"1234",
"qwertyuiop",
"123321",
"password123"
];
And If you notice the HTML markup inside the index.html
file, there is a <ul>
element with the ID of unsafe-passwords
.
Your goal is to go through each item inside the unsafePasswords
array using a "for" loop and insert that item as a <li>
item inside the <ul>
element with the ID of unsafe-passwords
:
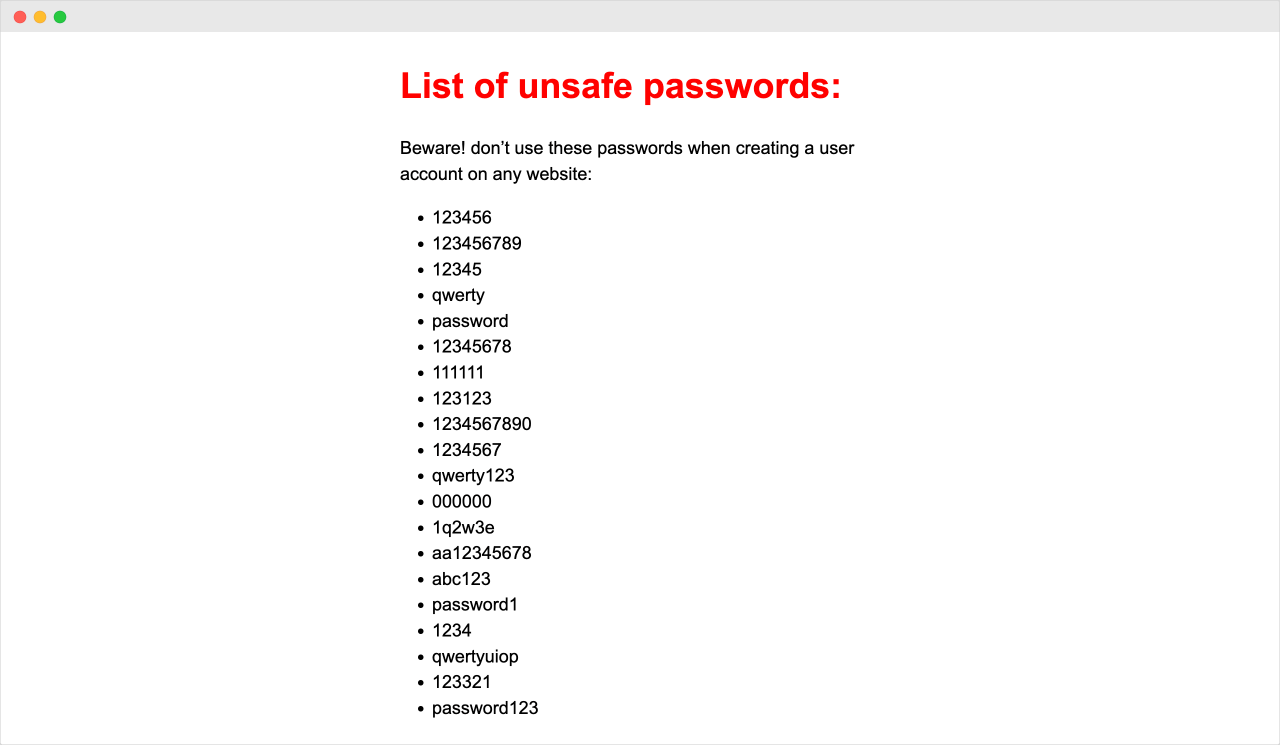
Also, for initializing the counter of the "for" loop, during the last few lessons, we created a variable and named it counter
:
for(let counter = 0; counter < 5; counter++ ){
console.log(unsafePasswords[counter]);
}
But this time, change the name of the counter variable to i
because if you start reading code on the internet, the counter variable is usually named as i
.
for(let i = 0; i < 5; i++ ){
console.log(unsafePasswords[counter]);
}
Get habituated to it, and if you're searching for its meaning, depending on the context of the loop, i
could mean an "index" or "iteration", or the developer is just lazy enough to give a meaningful name to the counter variable.
You ready?
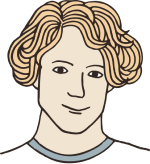
Yep! I am! It's Jujubi :D
Great!
...
...
...
The Solution
Here is the final javascript code to achieve our goal with an explanation via comments:
//Step 1: We have already created a list of unsafe passwords using an array
let unsafePasswords = [
"123456",
"123456789",
"12345",
"qwerty",
"password",
"12345678",
"111111",
"123123",
"1234567890",
"1234567",
"qwerty123",
"000000",
"1q2w3e",
"aa12345678",
"abc123",
"password1",
"1234",
"qwertyuiop",
"123321",
"password123"
];
//Step 2: Select the UL element where we will be placing each item from the above array.
const ulElement = document.querySelector("#unsafe-passwords");
//Step 3: Loop over each item from the array and output them to the web page by creating a LI element for each item.
for(let i = 0; i < unsafePasswords.length; i++){
//Create a new LI element for the current password
const liElement = document.createElement("li");
//Assign the text of the current password as content to the newly created LI element
liElement.textContent = unsafePasswords[i];
//Insert the newly created LI element inside the UL element.
ulElement.appendChild(liElement);
}
By the time the above loop stops running, all the items of the array are now present as LI elements inside the selected UL element:
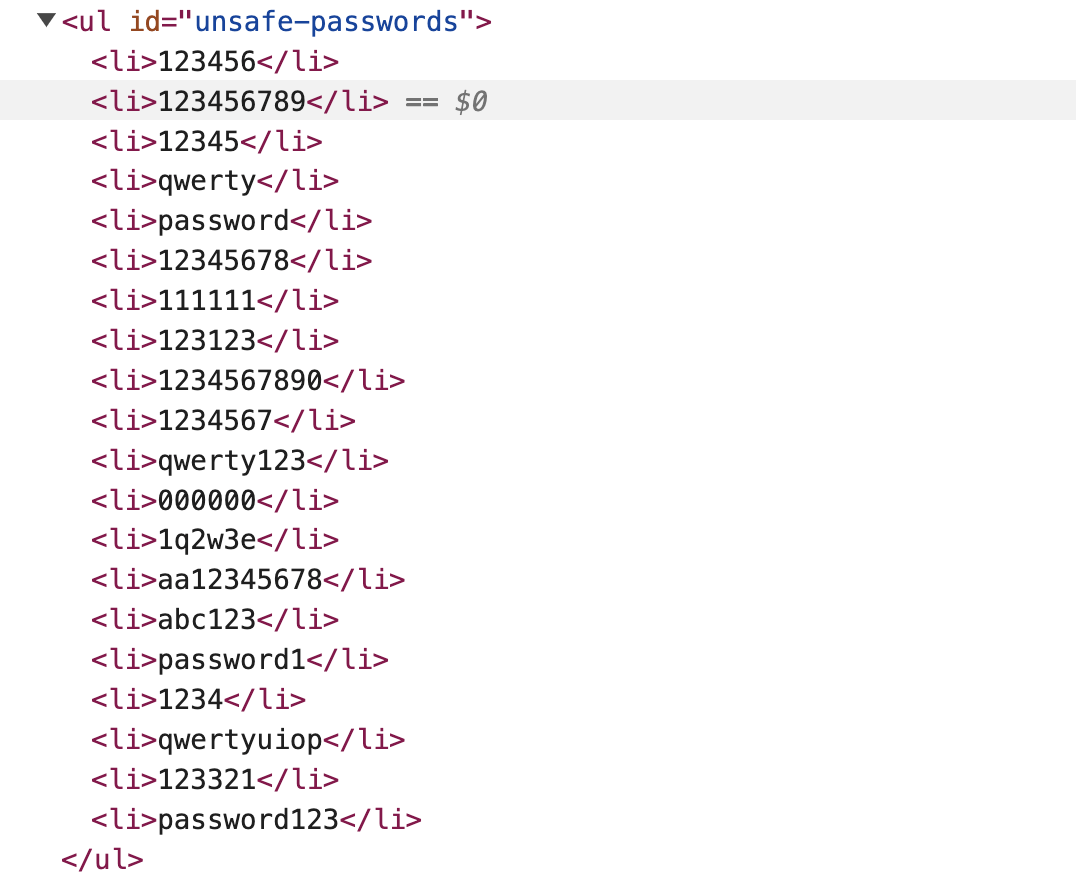
Just to be on the same page, here is what's happening step by step...
Before the loop
We have an array unsafePasswords
containing weak passwords and selected the <ul>
element where we will be placing each item from the array.
let unsafePasswords = [
"123456",
"123456789",
"12345",
"qwerty",
"password",
"12345678",
"111111",
"123123",
"1234567890",
"1234567",
"qwerty123",
"000000",
"1q2w3e",
"aa12345678",
"abc123",
"password1",
"1234",
"qwertyuiop",
"123321",
"password123"
];
const ulElement = document.querySelector("#unsafe-passwords");
Next, we have used a "for" loop to iterate over each item inside the unsafePasswords
array and output it as an <li>
element inside the ul
element we selected previously:
for(let i = 0; i < unsafePasswords.length; i++){
const liElement = document.createElement("li");
liElement.textContent = unsafePasswords[i];
ulElement.appendChild(liElement);
}
To be more clear, here is what's happening during each iteration...
Iteration 1 (i = 0):
- i is 0: The counter starts at 0, pointing to the first element in the array.
- Create liElement: A new list item (
<li>
) element is created and stored inliElement
variable. - Set liElement text: The text content of the new
li
element is set to the password at index 0 of the array, which is "123456" (the first password). - Append to ulElement: The newly created
liElement
with the password "123456" is added as a child of theulElement
. This shows "123456" as the first item in the "Unsafe Passwords" list on the web page.
Iteration 2 (i = 1):
- i is 1: The counter moves to 1, pointing to the second element in the array.
- Create liElement (new one): Remember,
liElement
is a variable, not the actual element. So, a new<li>
element is created and stored in the sameliElement
variable. - Set liElement text: The text content is now set to the password at index 1, which is "123456789" (the second password).
- Append to ulElement: This
liElement
with the password "123456789" is appended to theulElement
, adding it as the second item in the list on the web page.
Iteration 3 (i = 2) and so on:
- The loop continues similarly, incrementing
i
each time, creating a newliElement
for each password, setting its text content to the current password, and appending it to theulElement
. - After all iterations (until
i
reaches the array length), all passwords from the array will be displayed as separate list items within the "Unsafe Passwords" list.
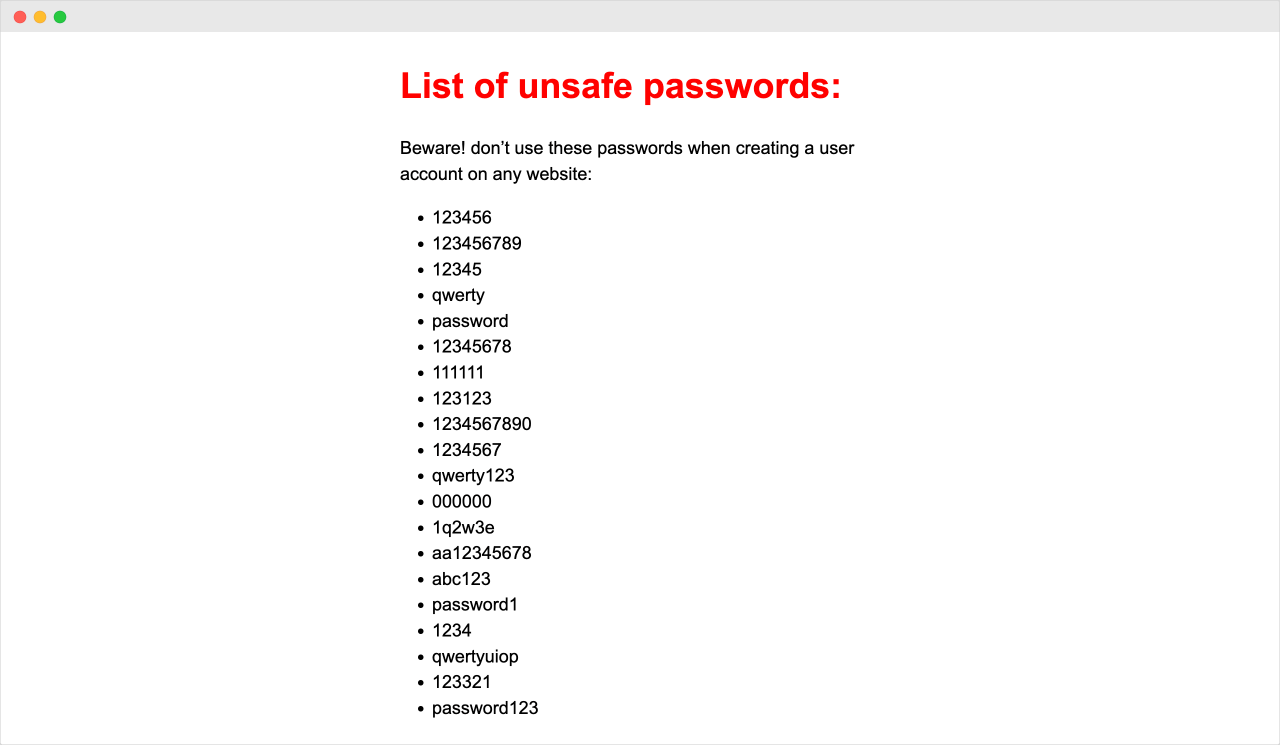
In the next lesson, we will talk about performance when dealing with loops.