Challenge: Convert a simple "for" loop to use a forEach method
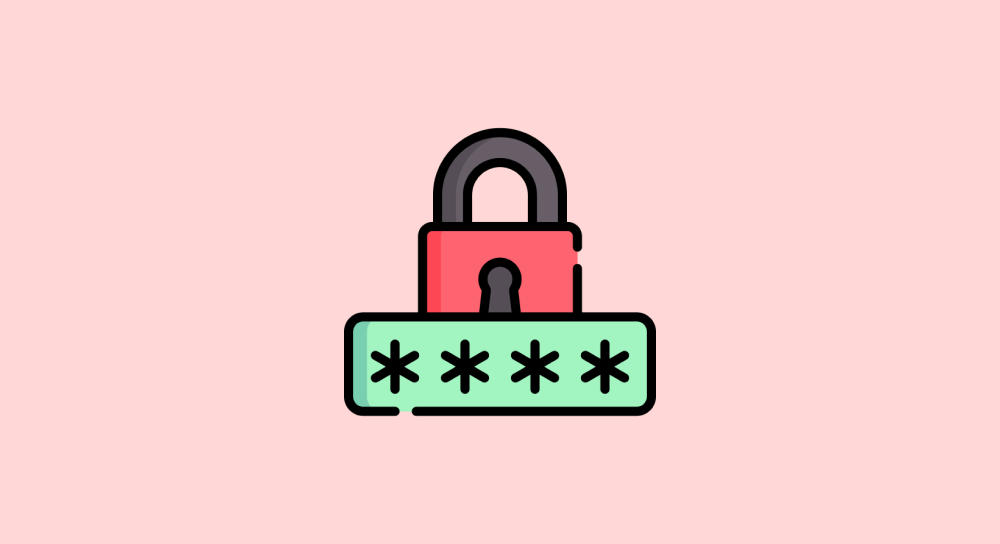
We are back to our boring "Output unsafe passwords to the web page" project again 🙈
I promise this is the last time we will work on this project. Once we understand NodeLists in the next lesson, we will work on real-world projects.
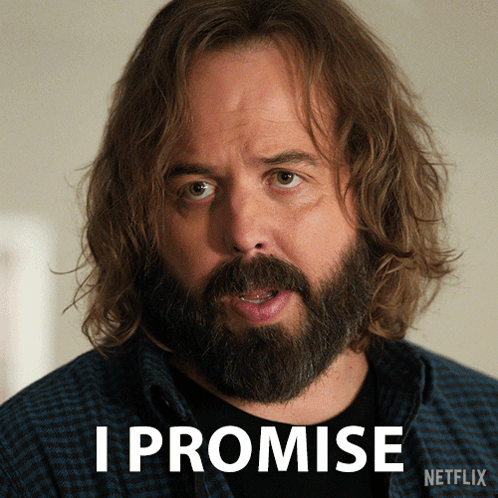
Anyway, download the project files and open them up in your code editor:
Next, if you open up the index.js
file, we are currently outputting the unsafe passwords using a "for" loop:
let unsafePasswords = [
"123456",
"123456789",
"12345",
"qwerty",
"password",
"12345678",
"111111",
"123123",
"1234567890",
"1234567",
"qwerty123",
"000000",
"1q2w3e",
"aa12345678",
"abc123",
"password1",
"1234",
"qwertyuiop",
"123321",
"password123"
];
//Select the parent element
const olElement = document.querySelector("#unsafe-passwords");
for(let i = 0; i < unsafePasswords.length; i++){
const liElement = document.createElement("li");
liElement.textContent = unsafePasswords[i];
olElement.appendChild(liElement);
}
Your job is simple. Replace the "for" loop with a "forEach" loop.
Come on, go ahead. I hate laziness 😅
The Solution
let unsafePasswords = [
"123456",
"123456789",
"12345",
"qwerty",
"password",
"12345678",
"111111",
"123123",
"1234567890",
"1234567",
"qwerty123",
"000000",
"1q2w3e",
"aa12345678",
"abc123",
"password1",
"1234",
"qwertyuiop",
"123321",
"password123"
];
//Select the parent element
const olElement = document.querySelector("#unsafe-passwords");
unsafePasswords.forEach(function(unsafePassword){
const liElement = document.createElement("li");
liElement.textContent = unsafePassword;
olElement.appendChild(liElement);
});
Easy enough, right?
And as promised, we will understand NodeLists in the next lesson.