Secret: An array is a specialized object
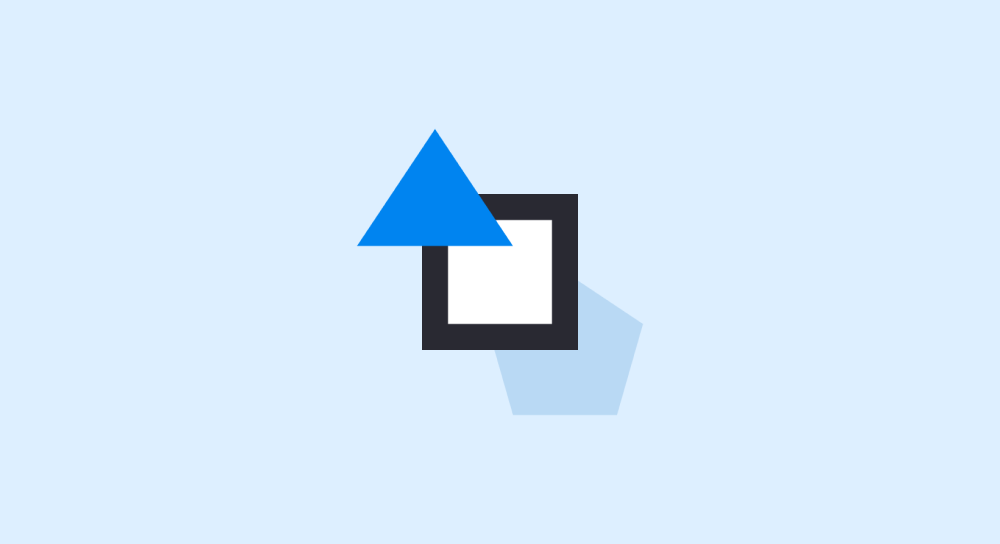
The problem:
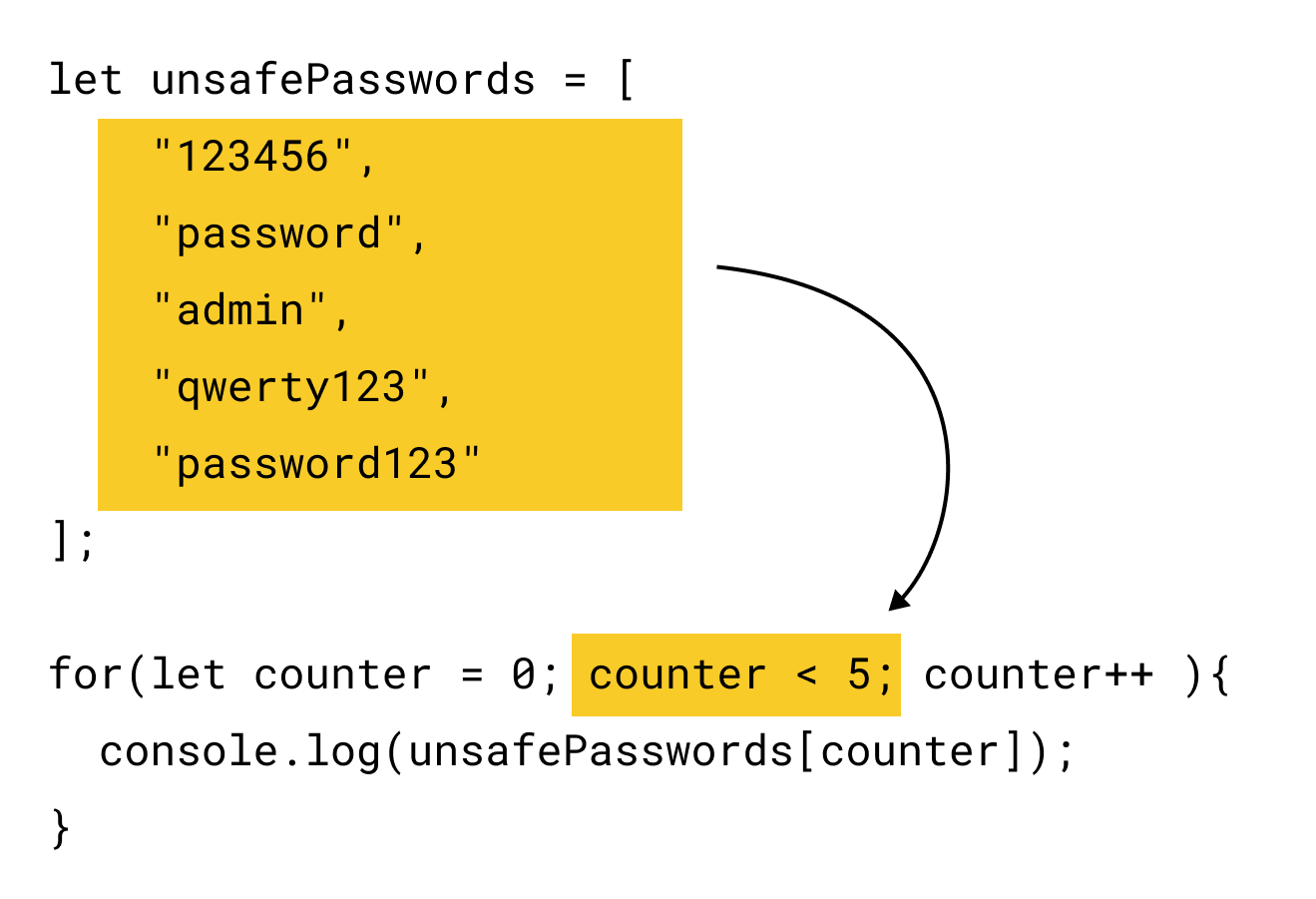
If you notice the conditional statement of the loop in the above diagram, we have provided the length of an array manually.
We did so:
- By manually counting the array length using our own brain
- And then by manually typing the array length
5
inside the conditional statementcount < 5
.
But when we are using automation, we shouldn't count the array length by ourselves, nor should we manually type it.
What if you don't know the length of the array beforehand? What if there are so many items that you can't count by yourself?
This is more than common when we get data from somewhere else on the internet.
How do we fix this problem?
Simple.
Whenever we create an array, it provides us with a length
property:
array.length;
When you call the length property on an array, it will return the count of the total number of items inside the array.
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
unsafePasswords.length; // It returns: 5
For example, if you notice the above snippet, the unsafePasswords
array has five items stored in it.
So, calling the length
property on the unsafePasswords array will return the value of 5.
And this length
property is especially useful if we want to perform some action on an array automatically and still run the loop only a certain number of times.
let unsafePasswords = [
"123456",
"password",
"admin",
"qwerty123",
"password123"
];
for(let counter = 0; counter <a unsafePasswords.length; counter++ ){
console.log(unsafePasswords[counter]);
}
If you notice the conditional statement of the above loop, this time, we are not providing the length of the array manually.
We are getting the lengthprogrammatically by using the length
property on the array:
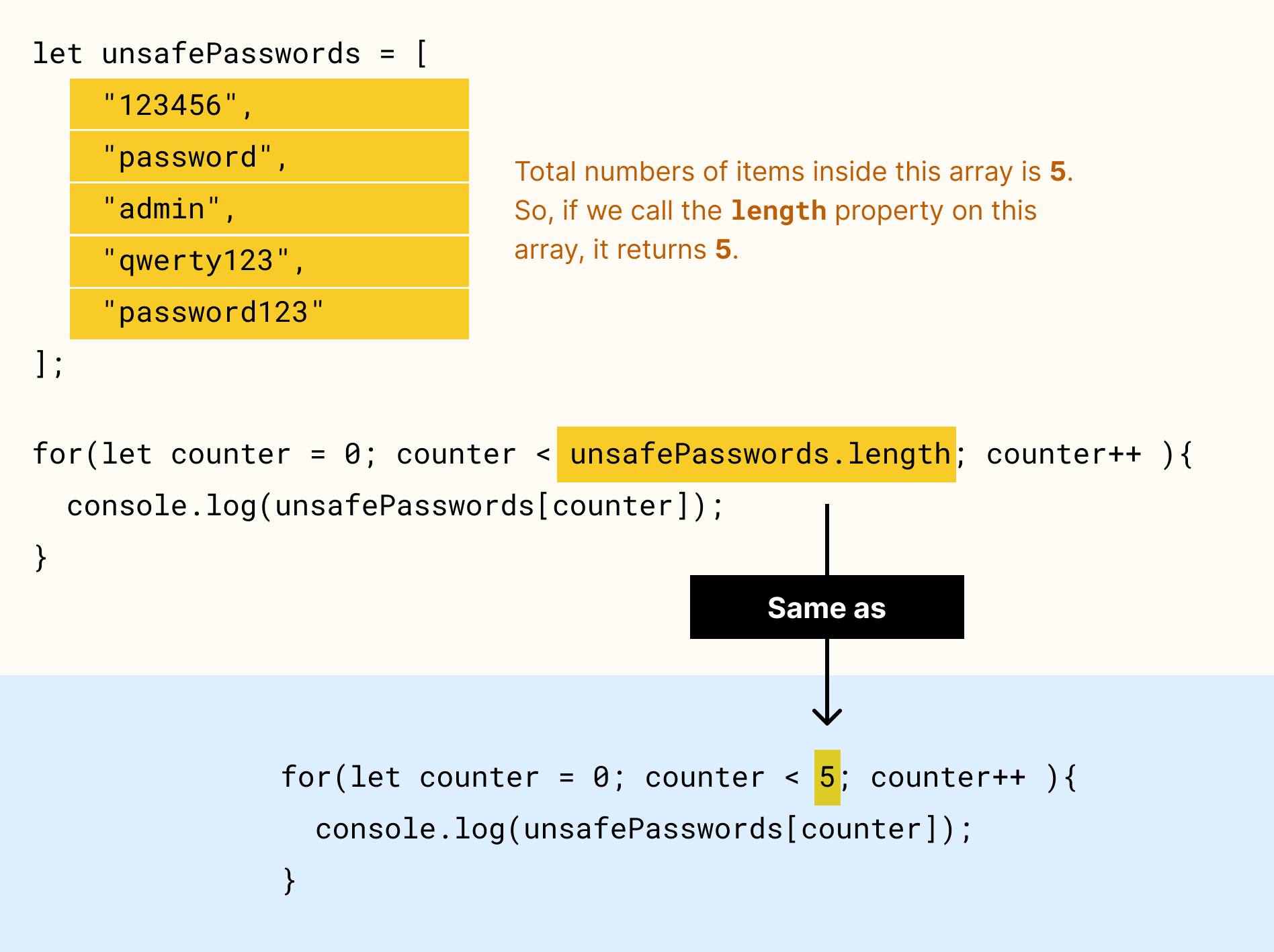
In other words, the following loop:
for(let counter = 0; counter < 5; counter++ ){
console.log(unsafePasswords[counter]);
}
Is technically the same as:
for(let counter = 0; counter < unsafePasswords.length; counter++ ){
console.log(unsafePasswords[counter]);
}
But without you providing the array length manually, you are getting the length automatically.
This makes our loop and code totally dynamic.
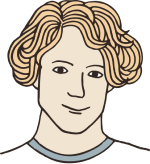
But bro! How can arrays have a length property?
Haha, as the title of this lesson says...
Arrays are a special kind of object
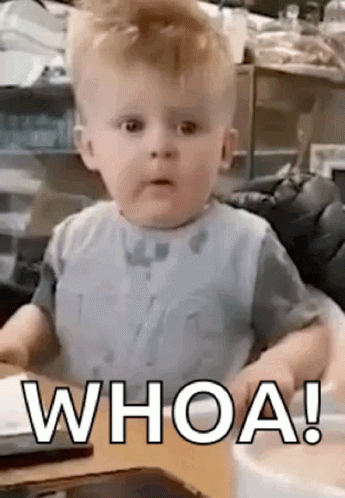
Simply put, arrays have all the features of regular objects plus additional features that make them work like arrays.
In other words:
- Just like an object, an array has properties and methods.
- Some of these properties and methods are specifically designed to work with items we store inside an array.
It is these methods and properties that make arrays flexible and helpful in managing a collection of items.
This is better practiced than explained.
practiced
let emojis = ["🌈", "🦄", "🤖", "🎃", "🍦", "🙈", "💡"];
Then, hit enter to finish creating the array.
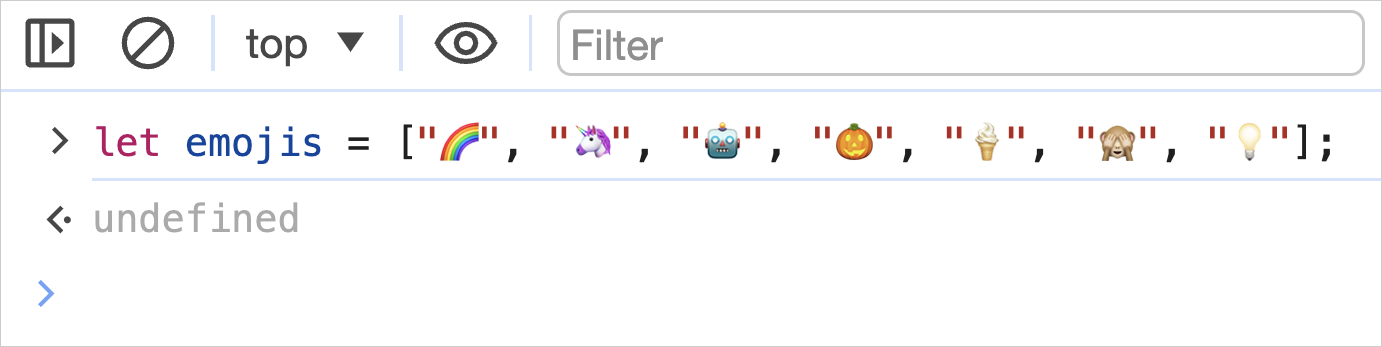
Next, we will explore some of the most commonly used properties and methods of arrays.
The "length" property
As you already know, it returns the count of the number of items inside an array.
For example, there are seven pieces of data inside the emojis
array:
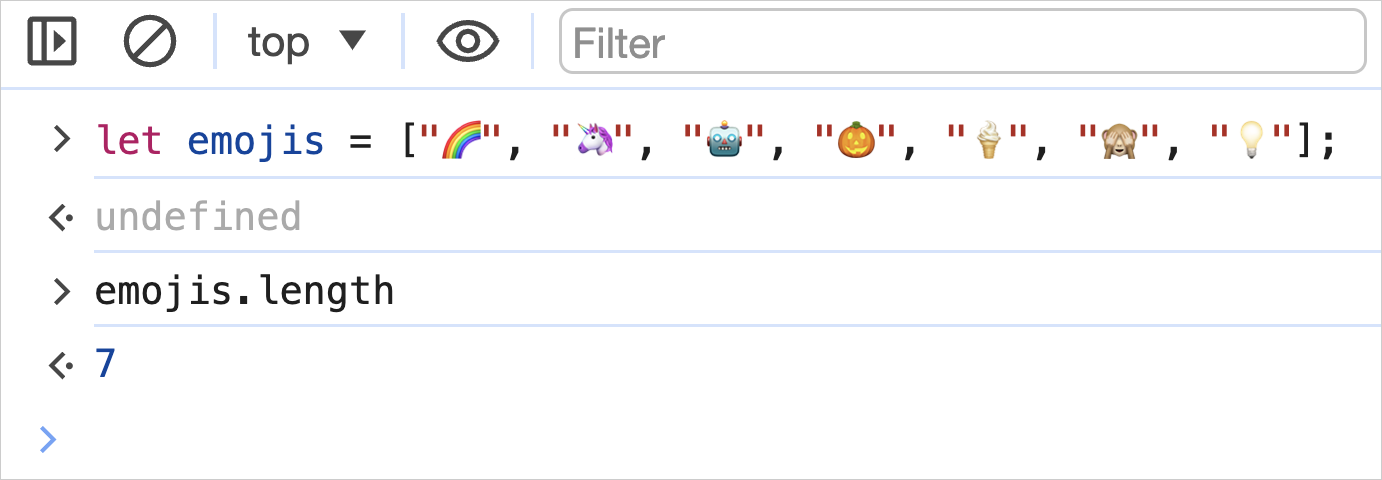
So, accessing emojis.length
property will give us 7
as the value.
The length
property is most helpful when iterating over arrays, as you have already seen.
An array is flexible. Once created, you can delete items from it or add new items to it anytime.
How to add an item to an array
You can achieve this with the help of the push()
method.
let emojis = ["🌈", "🦄", "🤖", "🎃", "🍦", "🙈", "💡"];
emojis.push("😈");
The push()
method adds the new item at the end of the array.
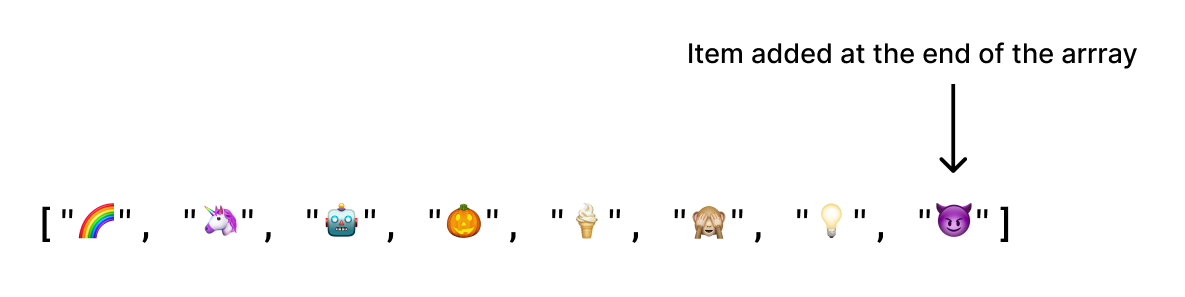
Also, remember that the push()
method changes the length of the array.
That means, if you now check the emojis.length
, it must be 8
. Previously it was 7
.
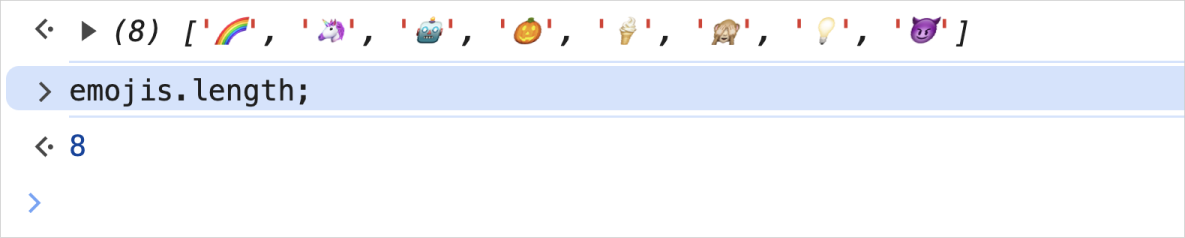
But what if you want to add an item to the beginning of the array?
Don't worry, the unshift()
method has got you covered.
The unshift()
method adds an item to the beginning of the array:
let emojis = ["🌈", "🦄", "🤖", "🎃", "🍦", "🙈", "💡", "😈"];
emojis.unshift("👽");
//Updated "emojis" array
["👽", "🌈", "🦄", "🤖", "🎃", "🍦", "🙈", "💡", "😈"]
I know, I know. The name unshift
doesn't make any sense at all 😵
But there is nothing much we can do about it.
Anyway, both push()
and unshift()
methods allow you to add multiple items to an array in a single go.
let emojis = ["🌈", "🦄", "🤖", "🎃", "🍦", "🙈", "💡", "😈"];
emojis.push("👹", "👺", "🤡");
//Updated array
["👽", "🌈", "🦄", "🤖", "🎃", "🍦", "🙈", "💡", "😈", "👹", "👺", "🤡"]
You can add any number of items you want. You're not limited to three.
How to delete an item from an array
You can achieve this with the help of the pop()
method.
The pop()
method removes the last element from an array and returns that element. This method changes the length of the array, too.
let emojis = ["👽", "🌈", "🦄", "🤖", "🎃", "🍦", "🙈", "💡", "😈"];
console.log(emojis.length); // Output: 9
// Remove the last item and save it to a variable
let removedElement = emojis.pop();
console.log(removedElement); // Outputs: 😈
console.log(emojis.length); // Output: 8
console.log(emojis);
// Outputs: ["👽", "🌈", "🦄", "🤖", "🎃", "🍦", "🙈", "💡"]
But what if you want to remove an item from the beginning of the array?
Don't worry. The shift()
method has got you covered.
The shift()
method removes an item from the beginning of the array:
let emojis = ["👽", "🌈", "🦄", "🤖", "🎃", "🍦", "🙈", "💡"];
console.log(emojis.length); // Output: 8
// Remove the first item and save it to a variable
let removedElement = emojis.shift();
console.log(removedElement); // Outputs: 👽
console.log(emojis.length); // Output: 7
console.log(emojis);
// Outputs: ["🌈", "🦄", "🤖", "🎃", "🍦", "🙈", "💡"]
push()
and unshift()
methods, the pop()
and shift()
methods can not remove multiple items at the same time. They can only remove one item at a time.There are a few other simple Array methods that I want to talk about.
But before that, let's work on a couple of small projects to cement the understanding of the "for" loops, arrays, and conditional statements.