Accessing the counter inside the loop body
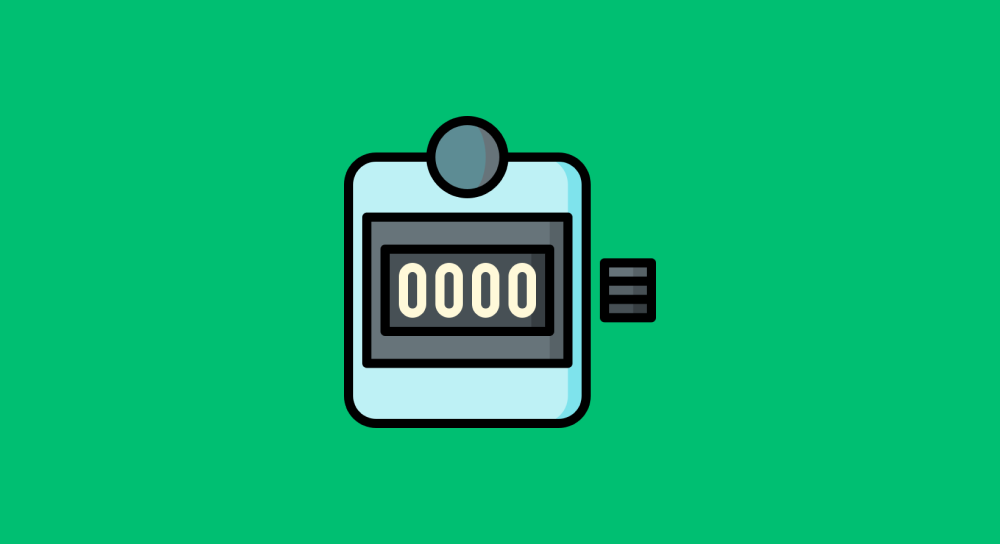
Now that you understand how a "for" loop works, it is time to show you something interesting.
Here is the loop from the previous lesson:
for( let counter = 1; counter <= 10; counter++ ){
console.log("Hello");
}
The thing is, sometimes, knowing the current value of the counter inside the loop body helps you achieve certain kinds of tasks.
And Javascript makes it really easy to do so:
for( let counter = 1; counter <= 10; counter++ ){
console.log("Hello");
console.log(counter);
}
If you notice the above snippet, we are directly accessing the current counter value by using its variable name counter
and then print it to the browser console:
console.log(counter);
Can you guess the output in the browser console?
...
...
...
Here you go:
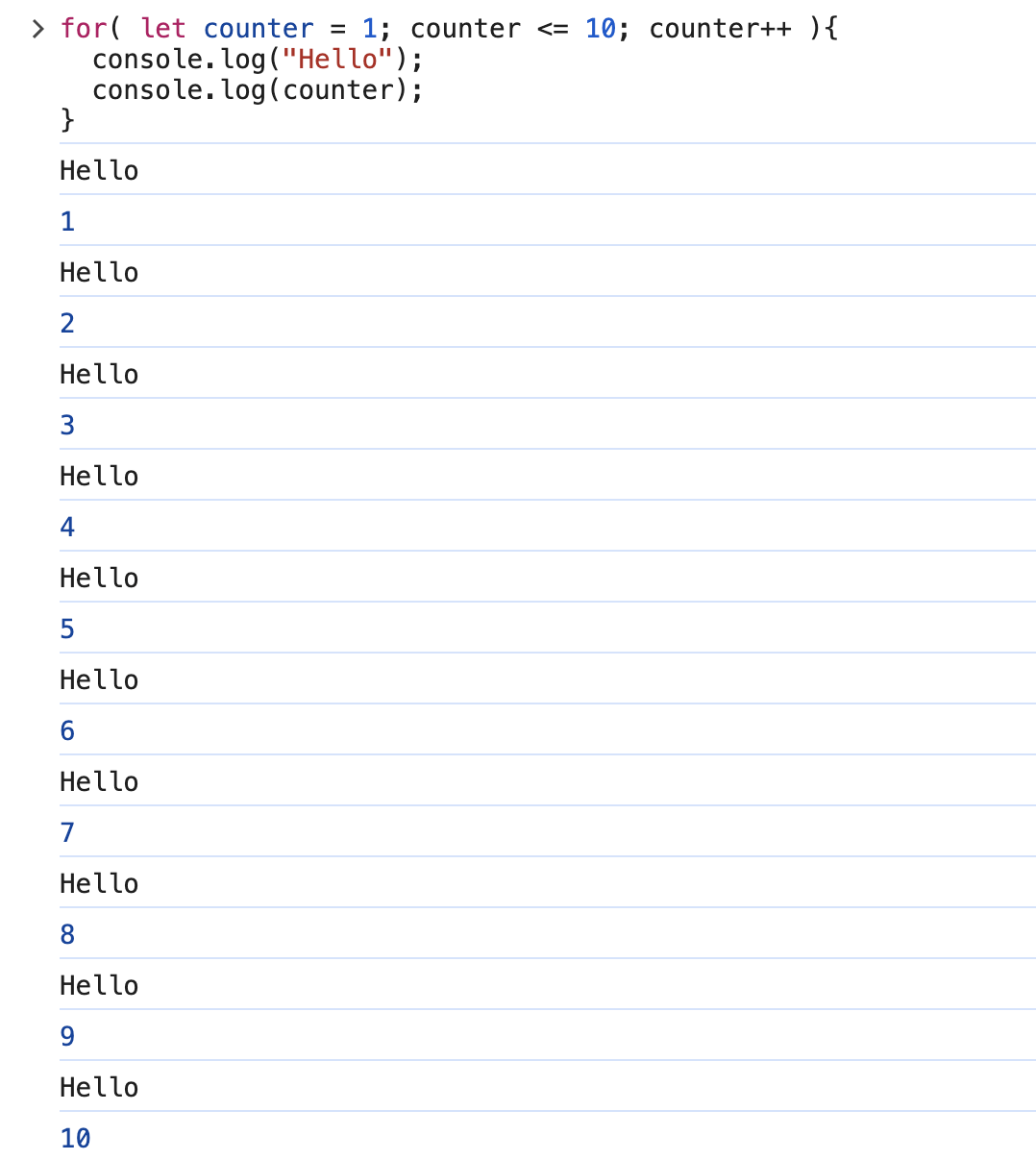
- During the first iteration (first loop cycle), the counter value is
1
, so,console.log(counter)
outputs1
to the browser console. - During the second iteration (second loop cycle), the counter value is updated to
2
because we have incremented it usingcounter++
, so,console.log(counter)
outputs2
to the browser console. - During the third iteration, the counter value is updated to
3
because we have incremented it usingcounter++
, so,console.log(counter)
outputs3
to the browser console.
You get the idea, right?
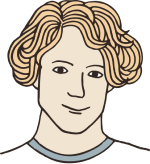
Yeah! But what's the purpose of accessing the counter value inside the loop? It is not clear for me.
Long story short, we usually write a "for" loop for accessing the items of an array in an automated way.
This will become clear in the next lesson :)